How to Efficiently Remove Duplicate Columns in Pandas?
Efficient Removal of Duplicate Columns in Pandas
When working with data in a dataframe, it's often necessary to remove duplicate columns to ensure data integrity and efficiency. In Pandas, there's an elegant solution to this problem.
Removing Duplicate Column Names
Suppose you have a dataframe with columns named 'Time', 'Time Relative', and multiple instances of 'Time'. To remove the duplicate column names, use the following code:
<code class="python">df = df.loc[:,~df.columns.duplicated()].copy()</code>
This approach checks for duplicate column names and retains only the unique ones.
Removing Duplicates Based on Values
In some cases, you may need to remove duplicate columns based on their values. The following code does just that:
<code class="python">df = df.loc[:,~df.apply(lambda x: x.duplicated(),axis=1).all()].copy()</code>
This code applies a lambda function to each column, checking for duplicated values. If all values in a column are unique, the column is kept; otherwise, it's discarded.
Note on Caveats
While the above approach efficiently removes duplicate columns based on values, it's crucial to consider specific use cases. Ensure that this method aligns with your data and desired outcome, as there might be situations where it may not provide the intended result.
By utilizing these approaches, you can effortlessly remove duplicate columns from your dataframe, ensuring data consistency and improving efficiency.
The above is the detailed content of How to Efficiently Remove Duplicate Columns in Pandas?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










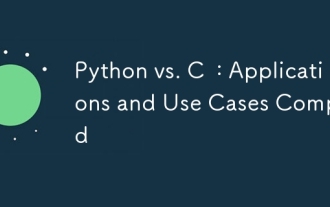
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
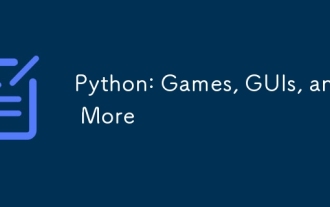
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
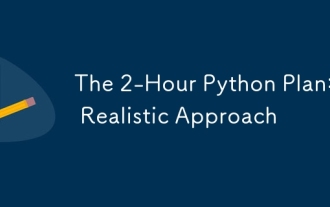
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
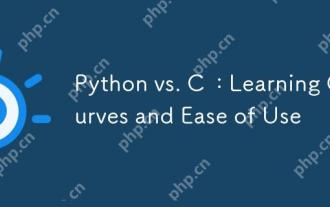
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
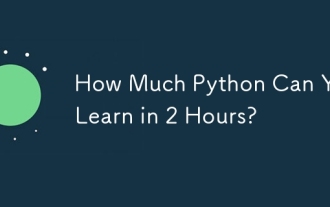
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
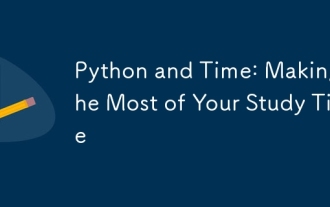
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
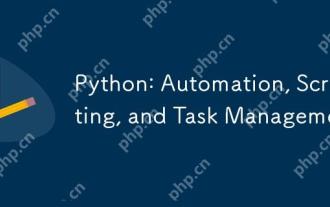
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
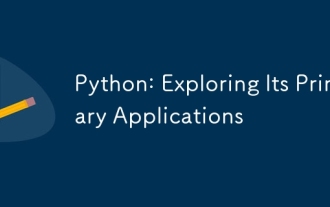
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
