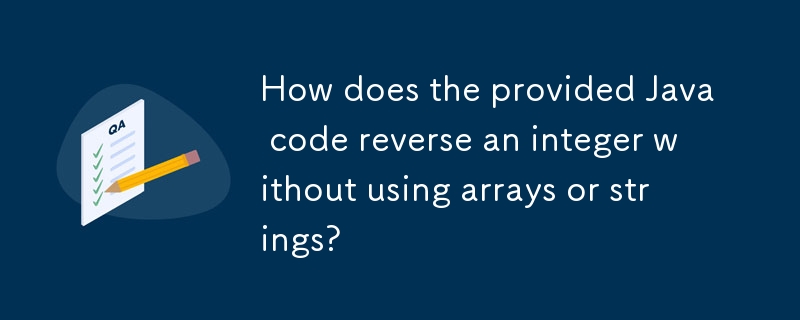
Reverse an Integer Without Arrays in Java
Your query concerns reversing an integer without recourse to arrays or strings. You encounter difficulties understanding the rationale behind the following code snippet:
1 2 3 4 | while (input != 0) {
reversedNum = reversedNum * 10 + input % 10;
input = input / 10;
}
|
Copy after login
Principles of Integer Reversal
To grasp the logic behind this algorithm, consider the following concepts:
-
Modulus (%): When input is divided by 10, the result is the last digit. (e.g., 1234 % 10 equals 4.)
-
Multiplication by 10: Multiplying an integer by 10 "shifts it left," adding a zero to its right. (e.g., 5 * 10 = 50.)
-
Division by 10: This operation removes the rightmost digit. (e.g., 75 / 10 = 7.)
Pseudocode
The reversal algorithm follows these steps:
- Extract the rightmost digit of the input.
- Append the extracted digit to the reversedNum variable.
- Multiply reversedNum by 10 to shift its digits to the left.
- Divide input by 10 to remove the examined digit.
- Repeat steps 1-4 until input reaches zero.
Working Code
Here is an updated version of the provided code that accommodates large input integers and handles possible overflow:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | <code class = "java" > public int reverseInt(int input) {
long reversedNum = 0;
long input_long = input;
while (input_long != 0) {
reversedNum = reversedNum * 10 + input_long % 10;
input_long = input_long / 10;
}
if (reversedNum > Integer.MAX_VALUE || reversedNum < Integer.MIN_VALUE) {
throw new IllegalArgumentException();
}
return (int) reversedNum;
}
|
Copy after login
Reverse Odd Numbers Only
To reverse only odd digits in an integer, you can implement the same algorithm but add a conditional statement to skip over even digits. For example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | public int reverseOddDigits(int input) {
long reversedNum = 0;
long input_long = input;
while (input_long != 0) {
if (input_long % 2 == 1) {
reversedNum = reversedNum * 10 + input_long % 10;
}
input_long = input_long / 10;
}
if (reversedNum > Integer.MAX_VALUE || reversedNum < Integer.MIN_VALUE) {
throw new IllegalArgumentException();
}
return (int) reversedNum;
}</code>
|
Copy after login
With this modified code, you can now selectively reverse the odd digits in your input integer.
The above is the detailed content of How does the provided Java code reverse an integer without using arrays or strings?. For more information, please follow other related articles on the PHP Chinese website!