


Can C 11 Lambdas Capture References Without Invoking Undefined Behavior?
Capturing References in C 11 Lambdas
In C , lambda expressions can capture variables from their enclosing scope. However, the method of capture determines whether the variable is accessed by reference or by value. Consider the following code:
<code class="cpp">#include <functional> #include <iostream> std::function<void()> make_function(int& x) { return [&]{ std::cout << x << std::endl; }; } int main() { int i = 3; auto f = make_function(i); i = 5; f(); }</code>
This code captures the variable x by reference using the [&] syntax. The question arises whether this program guarantees to output 5 without invoking undefined behavior.
Answer: Yes
The code is guaranteed to work correctly. Before examining the underlying standard wording, it is important to note that this code functions as intended by the C committee. However, the initial wording of the C 11 standard was unclear on this matter, leading to CWG issue 2011 being raised to provide clarification. This issue is being addressed in the ongoing development of the C standard.
Standard Explanation
According to [expr.prim.lambda]/17 of the C standard, only id-expressions referring to entities captured by copy are transformed into a member access on the lambda closure type. id-expressions referring to entities captured by reference are left alone and continue to denote the same entity they would have denoted in the enclosing scope.
In the code above, the captured entity is the parameter x of the make_function function, which falls within the reaching scope of the lambda. Therefore, the reference x in the lambda expression refers to the original variable declared in the main function.
It may initially appear problematic that x is being referenced outside its lifetime after the make_function function returns. However, there are limited scenarios in which a reference can be referenced outside its lifetime. Generally, a reference is either declared in scope or is a class member, in which case the class itself must be within its lifetime.
Therefore, the standard does not explicitly prohibit the use of references outside their lifetime. This loophole allowed for the capturing of references by reference in lambda expressions to be supported.
CWG Issue 2012 and Future Clarification
CWG issue 2012 was raised to address the oversight that references could be referenced outside their lifetime under certain circumstances. The resolution of this issue inadvertently affected the specification for lambda capture by reference of references. However, it is expected that this regression will be fixed before the release of C 17.
The above is the detailed content of Can C 11 Lambdas Capture References Without Invoking Undefined Behavior?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










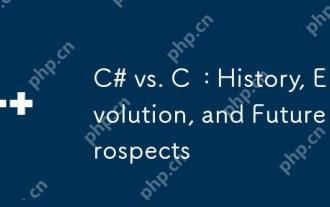
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
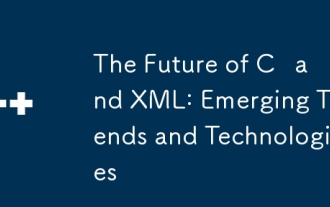
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
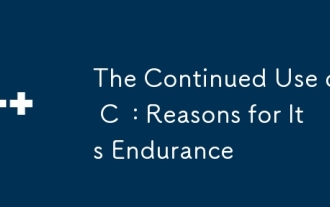
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
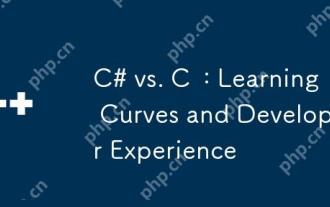
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
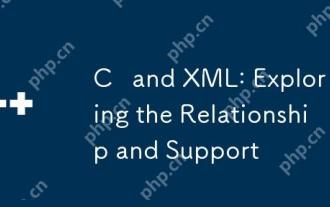
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
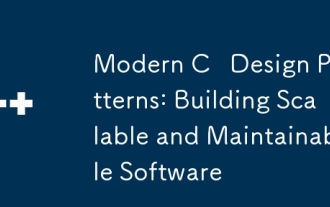
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
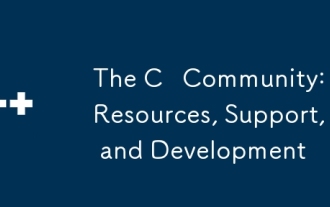
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
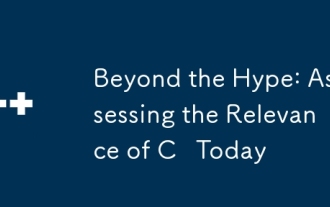
C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
