


How to Split a String Based on the First Occurrence of a Separator in Go?
Splitting Strings Based on First Element in Go
In Go, string splitting is commonly done using strings.Split() function. However, when dealing with strings containing multiple occurrences of a separator, you may encounter challenges in isolating the desired components.
Original Solution with Limitations
The provided example aimed to separate a string based on the first slash /. However, this approach faced issues when branch names also contained slashes.
Enhanced Solution: Iterating and Modifying Array
To address this, the solution was modified to take the first element of the split array, shift the remaining elements one position to the left, merge them back with a slash, and discard the last element. While this method worked, it lacked elegance.
Clean Solution: Using strings.SplitN()
For Go versions 1.18 and above, strings.SplitN() provides a more concise solution. It limits the split result to two substrings, ensuring the isolation of the remote and branch name components.
<code class="go">func ParseBranchname(branchString string) (remote, branchname string) { branchArray := strings.SplitN(branchString, "/", 2) remote = branchArray[0] branchname = branchArray[1] return }</code>
Advantages of strings.SplitN()
- Simplicity: It provides a straightforward and concise solution to the problem.
- Efficiency: By limiting the result to two substrings, it optimizes the splitting process.
- Extensibility: It can be easily adapted for different scenarios where a string needs to be split based on a specific number of separators.
The above is the detailed content of How to Split a String Based on the First Occurrence of a Separator in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










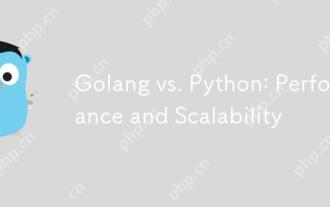
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
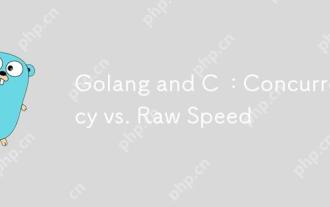
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
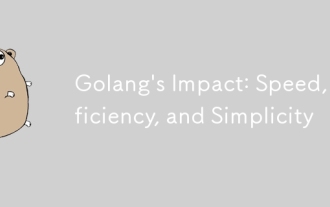
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
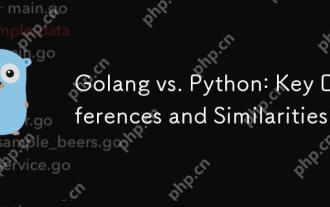
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
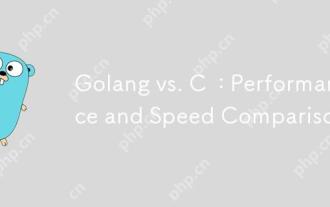
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
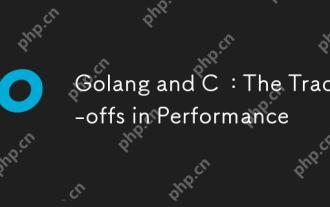
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
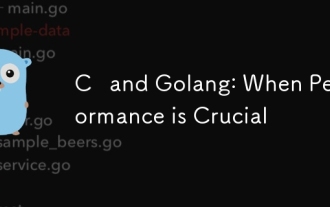
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
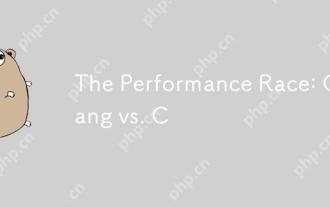
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
