


Why Does React Throw a 'Cannot Read Property 'setState' of Undefined' Error?
Understanding the "Cannot Read Property 'setState' of Undefined" Error in React
When working with React components, you may encounter the "Cannot read property 'setState' of undefined" error. This issue arises when attempting to access the setState method within a method of a React component, but the method has not been properly bound to the component instance.
Address the Binding Issue
In the provided example, this error occurs within the delta() method. The reason for this is that this.delta is not bound to the Counter component instance. To resolve this, use the following code in the constructor:
<code class="javascript">this.delta = this.delta.bind(this);</code>
Binding the delta method to the component instance ensures that it has access to the this context of the component, which allows it to access the setState method and the component state.
Updated Code
The corrected version of the code should look like this:
<code class="javascript">class Counter extends React.Component { constructor(props) { super(props); this.state = { count: 1, }; this.delta = this.delta.bind(this); // Bind 'delta' to the component instance } delta() { this.setState({ count: this.state.count++, }); } render() { return ( <div> <h1>{this.state.count}</h1> <button onClick={this.delta}>+</button> </div> ); } }</code>
By binding the delta method, you successfully resolve the "Cannot read property 'setState' of undefined" error and enable the component to increment the count as expected.
The above is the detailed content of Why Does React Throw a 'Cannot Read Property 'setState' of Undefined' Error?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
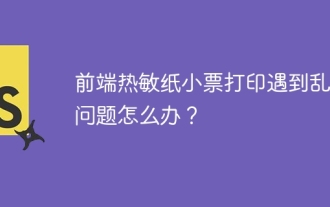
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
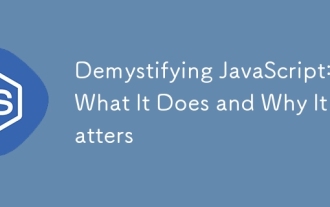
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
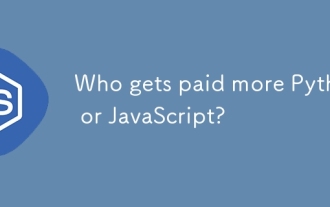
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
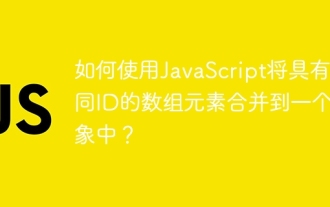
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
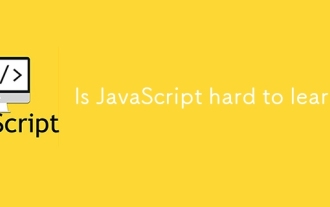
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
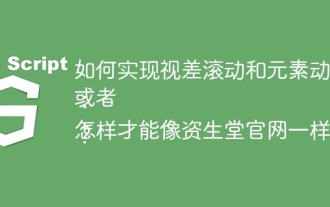
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
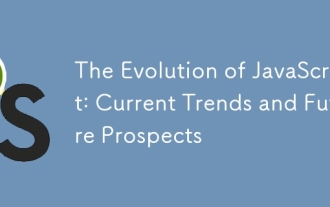
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
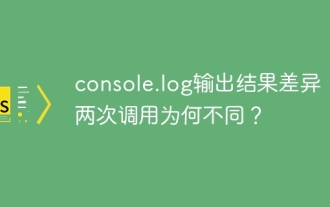
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
