


How can Jackson be used to convert JSON to Java objects, specifically when dealing with nested arrays?
Converting JSON to Java Objects with Jackson: A Guide
Decoding JSON into Java objects is a fundamental task in software development. This guide demonstrates how to effectively utilize Jackson to parse a complex JSON string that includes nested arrays.
Problem Statement
Given the following JSON string:
<code class="json">{ "libraryname": "My Library", "mymusic": [ { "Artist Name": "Aaron", "Song Name": "Beautiful" }, { "Artist Name": "Britney", "Song Name": "Oops I did It Again" }, { "Artist Name": "Britney", "Song Name": "Stronger" } ] }</code>
The goal is to convert this JSON into a Java object that allows easy access to nested values. Specifically, the desired object model enables retrieval of properties like:
- myobj.libraryname should return "My Library"
- myobj.mymusic[0].Song Name should return "Beautiful"
Jackson Solution
Jackson is a popular Java library for parsing and manipulating JSON. It provides two options for converting JSON to Java objects:
1. Map/List Approach
<code class="java">ObjectMapper mapper = new ObjectMapper(); Map<String, Object> map = mapper.readValue(jsonString, Map.class);</code>
This approach creates a simple Java map that mirrors the structure of the JSON. To access nested values, you can use the map's get method with the corresponding keys.
2. JSON Tree Approach
<code class="java">JsonNode rootNode = mapper.readTree(jsonString);</code>
Alternatively, you can create a JSON tree that represents the JSON structure. The JsonNode object provides methods like get and path for accessing and navigating through the tree.
POJO-Based Approach
For more convenient access to JSON values, you can define POJOs (Plain Old Java Objects) that match the structure of the JSON. Jackson can automatically map the JSON to these objects:
<code class="java">public class Library { private String libraryname; private List<Song> mymusic; } public class Song { private String artistName; private String songName; } Library lib = mapper.readValue(jsonString, Library.class);</code>
This approach provides strongly typed access to the JSON values and eliminates the need to cast or use generics.
Conclusion
Jackson provides a versatile and powerful solution for converting JSON to Java objects. Both the Map/List and POJO-based approaches offer flexibility and convenience depending on the specific requirements of your application.
The above is the detailed content of How can Jackson be used to convert JSON to Java objects, specifically when dealing with nested arrays?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










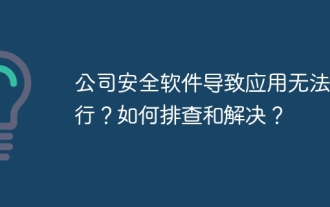
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
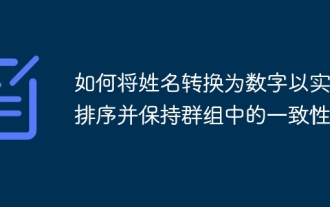
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
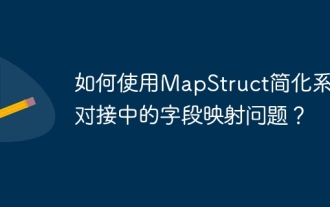
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
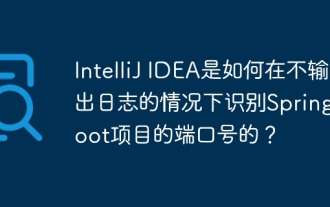
Start Spring using IntelliJIDEAUltimate version...
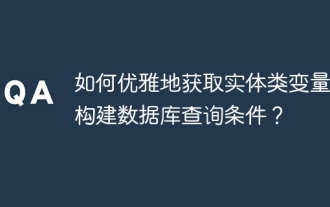
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
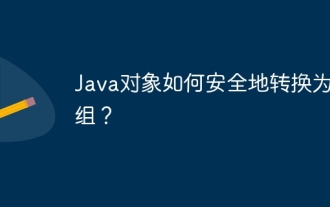
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
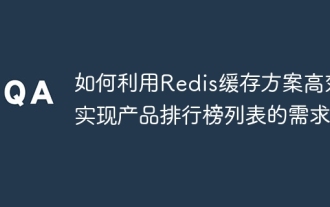
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
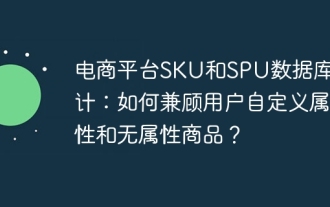
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
