How to Unmarshal JSON with Incorrect Timezone Offset in Go?
Invalid Datetime Format in JSON Unmarshalling
Background
Go's JSON unmarshaling has issues with datetimes formatted according to ISO8601/RFC3339 that have timezone offsets in the format 0200 instead of 02:00.
Problem
Incorrect JSON Format:
2016-08-08T21:35:14.052975+0200
Expected Correct Format:
2016-08-08T21:35:14.052975+02:00
Possible Cause:
The JSON was generated using C's strftime function with a format string that does not include the colon in the timezone offset.
Solution
To resolve this issue and enable the correct unmarshalling of both formats, a custom time field type can be defined:
<code class="go">type MyTime struct { time.Time } func (self *MyTime) UnmarshalJSON(b []byte) (err error) { s := string(b) // Remove double quotes from JSON value s = s[1:len(s)-1] t, err := time.Parse(time.RFC3339Nano, s) if err != nil { t, err = time.Parse("2006-01-02T15:04:05.999999999Z0700", s) } self.Time = t return }</code>
In this custom type, the UnmarshalJSON method attempts to parse the string according to both RFC3339Nano (with a colon in the timezone offset) and a modified version of RFC3339Nano without the colon.
Usage
To use the custom time field type:
<code class="go">type Test struct { Time MyTime `json:"time"` }</code>
This struct can then be unmarshalled from either JSON format with the incorrect or correct timezone offset.
Notes
- By default, the RFC3339Nano format in time.Parse uses "Z" for the timezone offset, while in the modified format, "Z0700" is used.
- The year "2006" in the time format is a reference to the first year of Go's release.
The above is the detailed content of How to Unmarshal JSON with Incorrect Timezone Offset in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
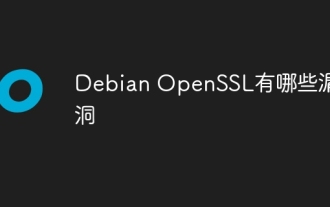
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
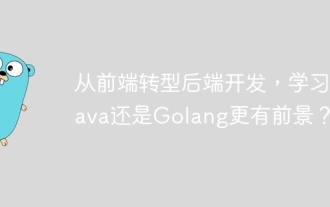
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
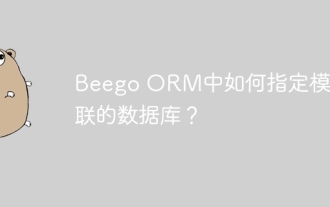
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
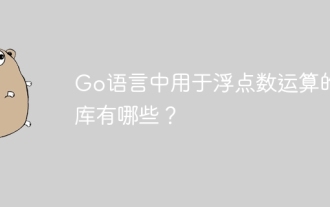
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
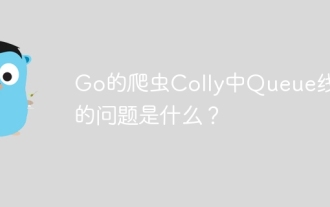
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
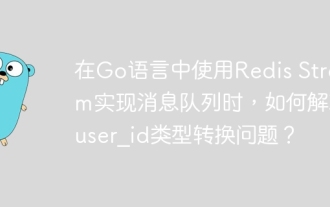
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
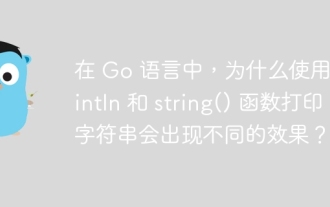
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
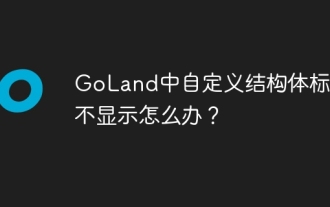
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
