


How to Unit Test Command Line Flag Values Against an Enumeration in Go?
Testing Command Line Flags in Go
This article explores testing techniques for command line flags in Golang. Specifically, we will examine how to unit test flag values against an enumeration.
Problem Statement
Given the following code:
<code class="go">// Define flag for output format var formatType string // Constants representing valid format types const ( text = "text" json = "json" hash = "hash" ) // Initialize flags func init() { flag.StringVar(&formatType, "format", "text", "Desired output format") } // Main function func main() { flag.Parse() }</code>
We wish to write a unit test to verify that the -format flag value matches one of the predefined constants.
Solution Using Custom Flag Type
To test flags in a more flexible manner, we can utilize the flag.Var function with a custom type implementing the Value interface.
<code class="go">package main import ( "errors" "flag" "fmt" ) // Custom type representing format type type formatType string // String() method for Value interface func (f *formatType) String() string { return fmt.Sprint(*f) } // Set() method for Value interface func (f *formatType) Set(value string) error { if len(*f) > 0 && *f != "text" { return errors.New("format flag already set") } if value != "text" && value != "json" && value != "hash" { return errors.New("Invalid Format Type") } *f = formatType(value) return nil } // Initialize flag with custom type func init() { typeFlag := "text" // Default value usage := `Format type. Must be "text", "json" or "hash". Defaults to "text".` flag.Var(&typeFlag, "format", usage) } // Main function func main() { flag.Parse() fmt.Println("Format type is", typeFlag) } </code>
In this solution, flag.Var takes a pointer to a custom type that satisfies the Value interface, allowing us to define our own validation logic within the Set method.
Unit Testing Custom Flag Type
Unit tests for the custom flag type can be written as follows:
<code class="go">// Test unit validates that the format flag is within the enumeration func TestFormatFlag(t *testing.T) { testCases := []struct { input string expectedErr string }{ {"text", ""}, {"json", ""}, {"hash", ""}, {"", "Invalid Format Type"}, {"xml", "Invalid Format Type"}, } for _, tc := range testCases { t.Run(tc.input, func(t *testing.T) { args := []string{"-format", tc.input} flag.CommandLine = flag.NewFlagSet("test", flag.PanicOnError) err := flag.CommandLine.Parse(args) if err != nil && err.Error() != tc.expectedErr { t.Errorf("Unexpected error: %v", err) return } }) } }</code>
The above is the detailed content of How to Unit Test Command Line Flag Values Against an Enumeration in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










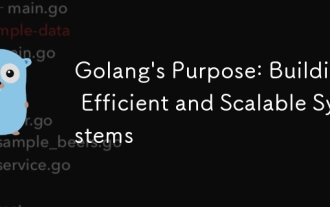
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
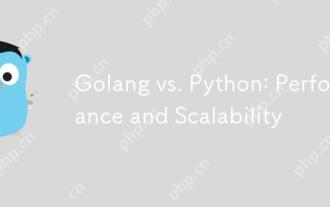
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
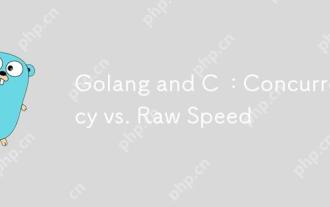
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
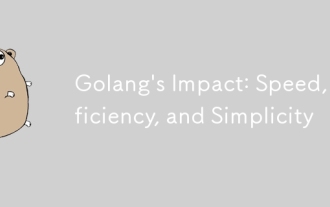
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
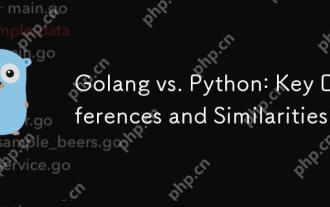
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
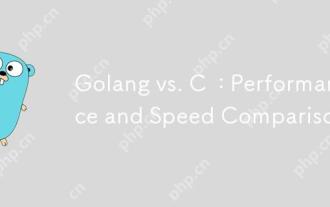
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
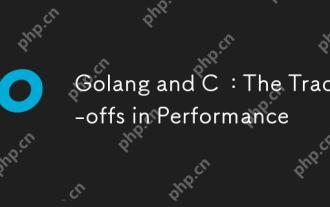
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
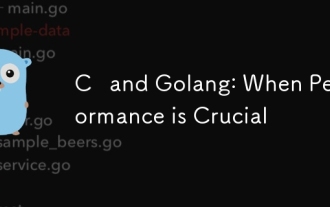
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
