


How to Achieve Natural Order Sorting in Python Like PHP's natsort?
Python's Equivalent to PHP's natsort for Natural Order Sorting
A frequently asked question in Python is how to sort a list in natural order, similar to PHP's natsort function. Unlike a standard sort, natural order considers numeric characters as numbers rather than strings.
For instance, consider sorting a list of filenames:
<code class="python">l = ['image1.jpg', 'image15.jpg', 'image12.jpg', 'image3.jpg'] l.sort()</code>
This standard sorting will result in:
['image1.jpg', 'image12.jpg', 'image15.jpg', 'image3.jpg']
However, to achieve a natural order sorting, Python provides a key function that converts strings into numeric tuples if possible. This allows for proper numeric comparisons.
Here's a sample implementation:
<code class="python">import re def natsort_key(s): return map(int, re.findall(r'(\d+)', s))</code>
Applying this key during sorting yields the desired natural order:
<code class="python">l.sort(key=natsort_key)</code>
The output will be:
['image1.jpg', 'image3.jpg', 'image12.jpg', 'image15.jpg']
Alternatively, if you require case-insensitive sorting, a more complete implementation can be used:
<code class="python">def natcasecmp(a, b): return natcmp(a.lower(), b.lower())</code>
This provides a case-insensitive natural order sorting.
The above is the detailed content of How to Achieve Natural Order Sorting in Python Like PHP's natsort?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










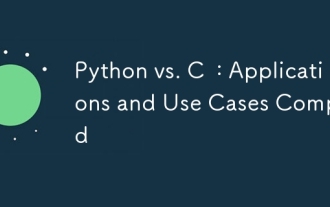
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
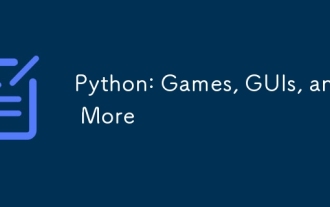
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
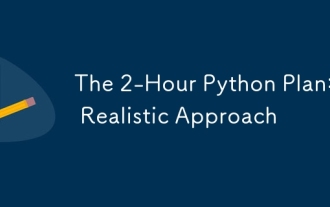
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
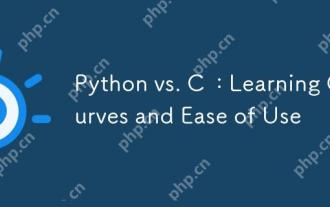
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
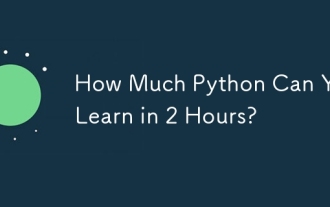
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
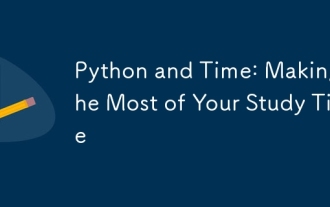
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
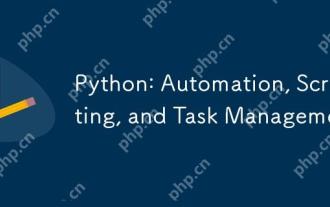
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
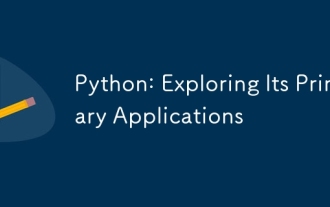
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
