How to Handle Binary Data in Go JSON Encoding?
Nov 05, 2024 pm 05:30 PMEncoding []byte Strings in JSON Using Go
Problem: Unexpected JSON Encoding of Binary Data
In Go, a []byte slice stores raw binary data. When attempting to encode a struct containing []byte fields into JSON using json.Marshal(), the resulting JSON contains an unexpected string representation of the slice contents instead of the original binary data. For example:
<code class="go">type Msg struct { Content []byte } func main() { msg := Msg{[]byte("Hello")} json, _ := json.Marshal(msg) fmt.Println(string(json)) // Prints {"Content":"SGVsbG8="} }</code>
Reason for Base64 Encoding
json.Marshal() encodes []byte slices as base64-encoded strings because JSON does not have a native representation for raw bytes. Base64 encoding represents binary data using a sequence of printable ASCII characters.
Solution: Recovering Original Binary Data
To retrieve the original binary data from the base64-encoded string in the JSON, simply decode the string using the base64.StdEncoding.DecodeString function:
<code class="go">import "encoding/base64" func main() { ... decodedBytes, _ := base64.StdEncoding.DecodeString(msg.Content) fmt.Println(string(decodedBytes)) // Prints "Hello" }</code>
The above is the detailed content of How to Handle Binary Data in Go JSON Encoding?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
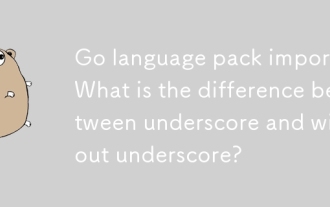
Go language pack import: What is the difference between underscore and without underscore?

How to implement short-term information transfer between pages in the Beego framework?
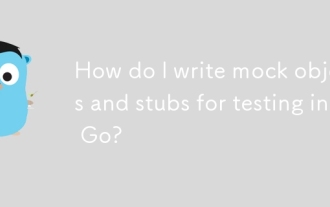
How do I write mock objects and stubs for testing in Go?
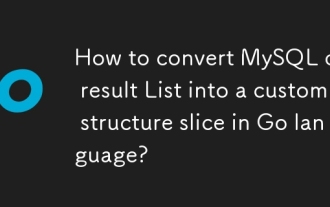
How to convert MySQL query result List into a custom structure slice in Go language?
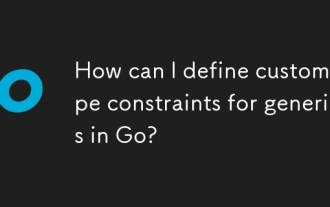
How can I define custom type constraints for generics in Go?
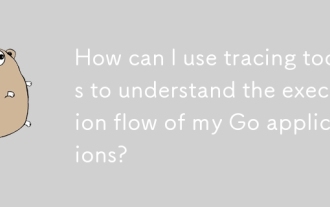
How can I use tracing tools to understand the execution flow of my Go applications?
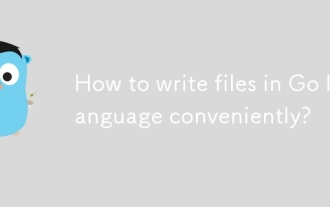
How to write files in Go language conveniently?
