


Why Do I Get a Syntax Error When Defining Nested Arguments in Python 3?
Python 3: Syntax Error While Defining Nested Arguments
Error Context
Developers may encounter a syntax error when compiling Python code into a module due to the attempted definition of nested arguments. While the same code runs seamlessly in IDLE using the "Run module" option, an error similar to the following may arise during distribution:
SyntaxError: invalid syntax File "/usr/local/lib/python3.2/dist-packages/simpletriple.py", line 9 def add(self, (sub, pred, obj)): ^
Cause
This error occurs because of the removal of tuple argument unpacking in Python 3 as explained in PEP 3113.
Solution
To rectify this error, the code should be modified to pass the tuple as a single parameter and unpack it manually. The affected code, def add(self, (sub, pred, obj)):, should be revised as follows:
def add(self, sub_pred_obj): sub, pred, obj = sub_pred_obj
For lambda functions, it is generally preferable to avoid unpacking altogether. Instead of using:
lambda (x, y): (y, x)
It is recommended to write:
lambda xy: (xy[1], xy[0])
Automated Tools
To facilitate the detection and correction of this issue, developers can utilize programs such as "2to3," "modernize," or "futurize" to refactor their Python 2.x code to Python 3.x, effectively identifying and suggesting suitable solutions for nested argument handling.
The above is the detailed content of Why Do I Get a Syntax Error When Defining Nested Arguments in Python 3?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




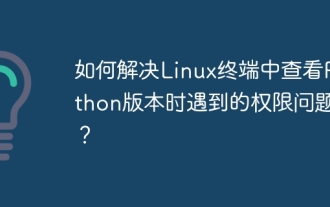
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
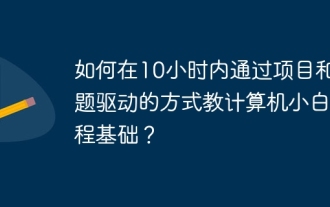
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
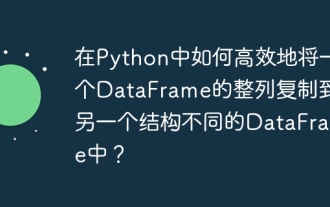
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
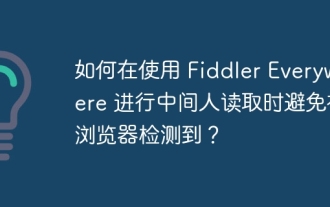
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
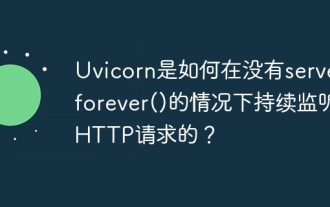
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
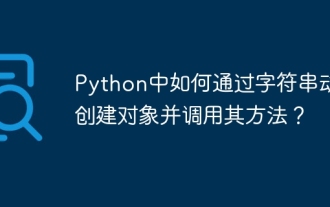
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
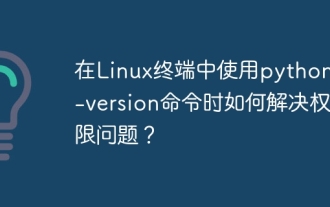
Using python in Linux terminal...
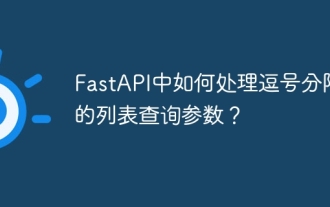
Fastapi ...
