How to Read Concurrently from Multiple Channels in Go?
Nov 05, 2024 pm 08:37 PMConcurrency and Channel Communication in Golang: Reading from Multiple Channels Simultaneously
In the realm of Golang, you may encounter the need to read from multiple channels concurrently. This specific query centers around constructing an 'any-to-one' channel setup, where two go-routines (numgen1 and numgen2) concurrently write numbers to separate channels (num1 and num2) and the goal is to aggregate these numbers in a third go-routine (addnum).
Consider the following:
<code class="go">func addnum(num1, num2, sum chan int) { done := make(chan bool) go func() { n1 := <-num1 done <- true }() n2 := <-num2 <-done sum <- (n1 + n2) }</code>
Issue:
Regrettably, the code snippet provided is flawed. It relies on a polling mechanism, and it is prone to deadlocks.
Solution:
A robust solution to this problem involves employing a select statement:
<code class="go">func main() { c1 := make(chan int) c2 := make(chan int) out := make(chan int) go func(in1, in2 <-chan int, out chan<- int) { for { select { case n1 := <-in1: out <- n1 case n2 := <-in2: out <- n2 } } }(c1, c2, out) }</code>
Explanation:
The select statement effectively blocks until data arrives on any of the input channels, essentially creating an any-to-one channel. As data becomes available, it is immediately sent through the out channel.
This method elegantly handles the situation where data may arrive on either channel at unpredictable intervals. It also eliminates the risk of deadlocks by constantly awaiting input from both sources.
Remember to utilize directional channel types as formal parameters for goroutine functions. This practice enhances code safety by ensuring that the compiler helps prevent improper channel usage.
With these insights, you can now confidently manage concurrent channel communication in Golang.
The above is the detailed content of How to Read Concurrently from Multiple Channels in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
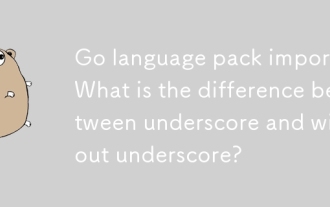
Go language pack import: What is the difference between underscore and without underscore?

How to implement short-term information transfer between pages in the Beego framework?
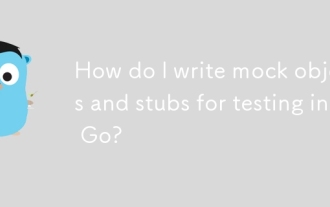
How do I write mock objects and stubs for testing in Go?
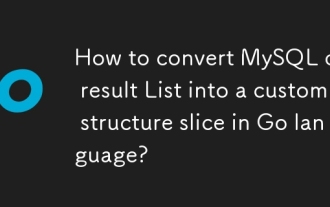
How to convert MySQL query result List into a custom structure slice in Go language?
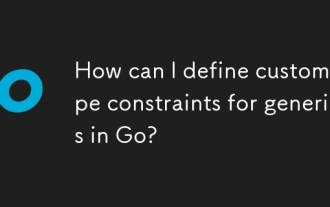
How can I define custom type constraints for generics in Go?
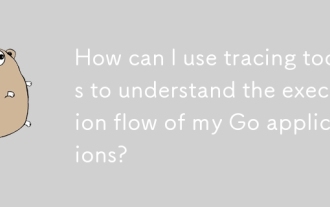
How can I use tracing tools to understand the execution flow of my Go applications?
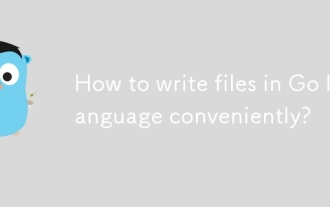
How to write files in Go language conveniently?
