


How to Resume Reading a File from a Specific Line Using the Scanner in Go?
Reading a File from a Specific Line Number Using Scanner
When working with files, it's often necessary to resume reading a file from a specific line number. The Scanner type in the bufio package can be extended to support this functionality.
Custom Split Function
The problem with using Scanner lies in its lack of line positioning capabilities. However, we can create a custom SplitFunc, which splits the input based on the newline character, and returns the count of characters read to maintain the file position.
func scanLinesWithPosition(data []byte, atEOF bool) (advance int, token []byte, err error) { advance, token, err = bufio.ScanLines(data, atEOF) pos += int64(advance) return }
This SplitFunc increments a position variable with the number of characters read.
Modified Scanner
Next, we need to modify the Scanner to use our custom SplitFunc.
func withScannerAtPosition(input io.ReadSeeker, start int64) error { fmt.Println("--SCANNER, start:", start) if _, err := input.Seek(start, 0); err != nil { return err } scanner := bufio.NewScanner(input) scanner.Split(scanLinesWithPosition) for scanner.Scan() { fmt.Printf("Pos: %d, Scanned: %s\n", pos, scanner.Text()) } return scanner.Err() }
Example Usage
In this example, we read a sample file and print its contents with line numbers:
func main() { input := strings.NewReader("first\r\nsecond\nthird\nfourth") if err := withScannerAtPosition(input, 0); err != nil { fmt.Println("Scanner error:", err) } }
Output:
--SCANNER, start: 0 Pos: 7, Scanned: first Pos: 14, Scanned: second Pos: 20, Scanned: third Pos: 26, Scanned: fourth
The above is the detailed content of How to Resume Reading a File from a Specific Line Using the Scanner in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










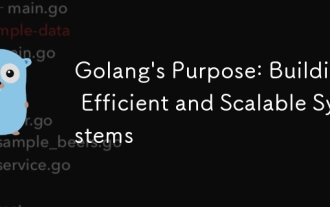
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
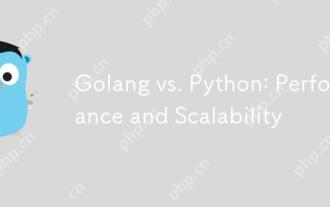
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
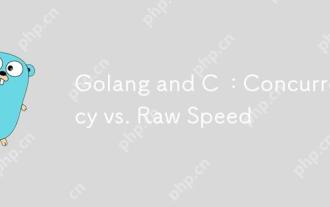
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
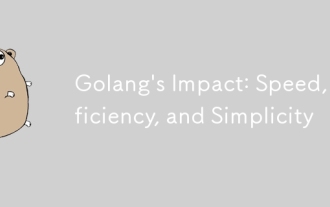
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
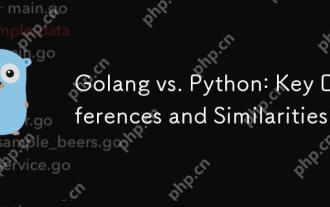
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
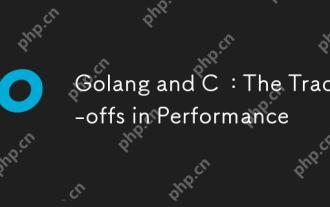
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
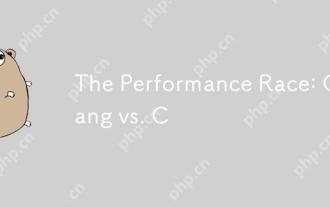
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
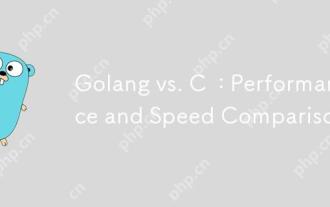
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
