How to Optimize Prime Number Mapping for a Limited Range?
Optimizing Prime Number Mapping for a Limited Range
Identifying prime numbers within a given range is a fundamental mathematical problem. The ultimate goal is to devise an algorithm that minimizes memory consumption while efficiently identifying primes for numbers up to a specified limit N.
Existing Approach: Bitmasking Odd Numbers
One approach for odd numbers is to use bitmasking, where each bit represents the prime status of a corresponding number. For example, the range (1, 10] would be represented as 1110, where the 1s indicate primes (3, 5, 7, 9).
Refining the Bitmask
However, this approach can be improved by eliminating multiples of five. For the given range, the revised bitmask becomes 11100. However, numbers ending in 1, 3, 7, or 9 still require individual bits.
Optimal Solution
The most compact algorithm for this specific problem varies depending on the range and available computational resources.
- AKS Algorithm: AKS is the most efficient algorithm for general prime testing. However, it is computationally expensive for large ranges.
- Special Primes: For large ranges, consider finding primes with specific forms, such as Mersenne primes.
- Python Implementation: For limited ranges, a variant of the O(sqrt(N)) algorithm can be used:
<code class="python">def isprime(n): if n == 2: return True if n == 3: return True if n % 2 == 0: return False if n % 3 == 0: return False i = 5 w = 2 while i * i <= n: if n % i == 0: return False i += w w = 6 - w return True</code>
Additional Optimizations
- Fermat's Pseudo-Prime Test: For restricted ranges, this test can provide significant speed improvements.
- Precomputing False Positives: By identifying numbers that satisfy Fermat's theorem but are not prime (Carmichael numbers), a binary search can be used for even faster testing.
The specific optimization strategy depends on the desired performance and memory constraints for the particular range of numbers being considered.
The above is the detailed content of How to Optimize Prime Number Mapping for a Limited Range?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










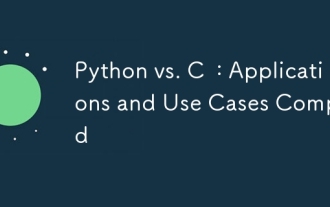
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
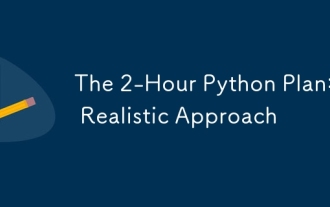
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
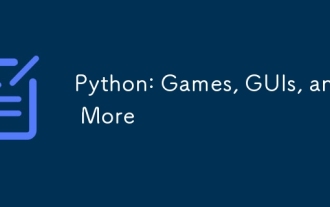
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
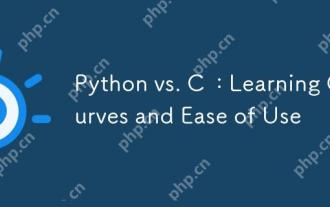
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
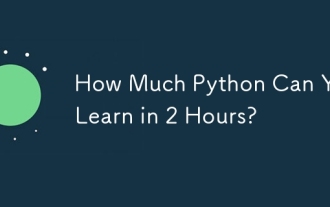
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
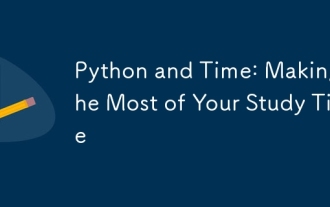
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
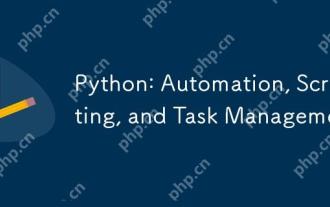
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
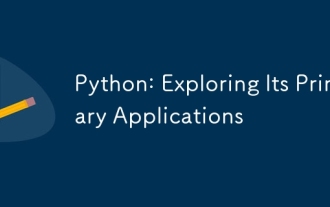
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
