


How to Search for Multiple Keywords Across Multiple Columns with Relevance-Based Ordering in Laravel?
Nov 06, 2024 pm 02:09 PMLaravel: Searching Multiple Keywords Against Multiple Columns with Relevance-Based Ordering
In Laravel, implementing a search that considers multiple keywords against multiple columns while maintaining relevance can be challenging. This article addresses such a problem, where the search results are ordered based on the frequency of the keywords' occurrence in the specified columns.
Scenario:
The search bar requires three keywords as input, and the search criteria is:
- Rows containing all three keywords in both columns (meta_name and meta_description) come first.
- Rows containing only the first two keywords in the mentioned columns come second.
- Rows containing only the first keyword in the mentioned columns come third.
Database Query:
To achieve the desired result, the database query should combine multiple OR conditions and utilize the whereNotIn function to exclude rows that have already been fetched in previous conditions.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
|
Union and Ordering:
Finally, the $all, $twoWords, and $oneWord results are merged using the union function to obtain the ordered search results.
1 2 3 4 5 |
|
This approach ensures that the search results are ordered as per the specified criteria, starting with rows containing all three keywords in the specified columns.
The above is the detailed content of How to Search for Multiple Keywords Across Multiple Columns with Relevance-Based Ordering in Laravel?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
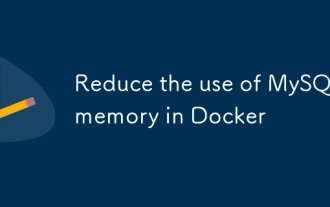
Reduce the use of MySQL memory in Docker
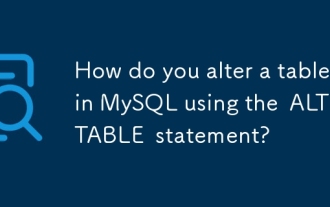
How do you alter a table in MySQL using the ALTER TABLE statement?
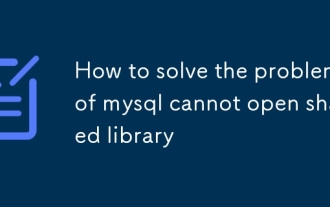
How to solve the problem of mysql cannot open shared library
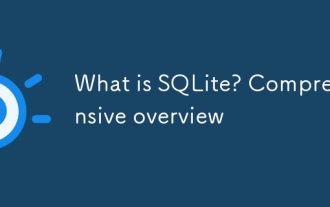
What is SQLite? Comprehensive overview
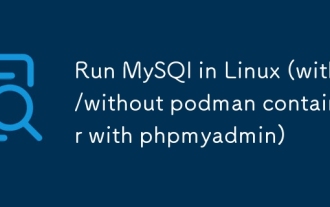
Run MySQl in Linux (with/without podman container with phpmyadmin)
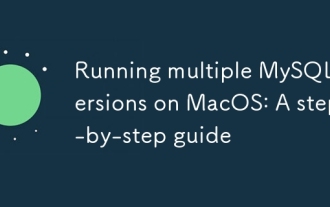
Running multiple MySQL versions on MacOS: A step-by-step guide
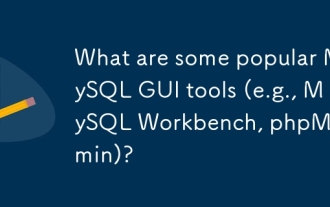
What are some popular MySQL GUI tools (e.g., MySQL Workbench, phpMyAdmin)?
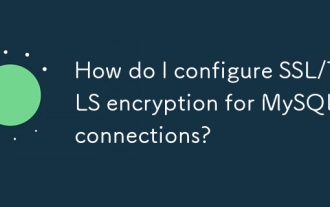
How do I configure SSL/TLS encryption for MySQL connections?
