How to Detect Signed Integer Overflow in Go?
Detecting Signed Integer Overflow in Go
Integer overflow is a crucial issue to consider when working with numeric operations in any programming language, including Go. In this context, understanding how to efficiently detect integer overflow is essential to ensure data integrity and avoid unexpected behavior.
For 32-bit signed integers, Overflow can occur when the result of an arithmetic operation exceeds the maximum or minimum representable value for that type. A common misconception is that integers automatically "wrap" around when they reach their limits, but this is not the case in Go. Instead, integer overflow results in undefined behavior.
To illustrate this problem, consider the following example:
a, b := 2147483647, 2147483647 c := a + b
If we naively perform this addition, the result will not be the expected value of 4294967294, but rather 0. This is because the sum overflows the maximum value that a 32-bit signed integer can hold. Therefore, it is important to detect such overflows explicitly.
One efficient way to detect 32-bit integer overflow for addition is by checking the signs of the operands and the result. For instance, the following function performs this check for addition:
func Add32(left, right int32) (int32, error) { if right > 0 { if left > math.MaxInt32-right { return 0, ErrOverflow } } else { if left < math.MinInt32-right { return 0, ErrOverflow } } return left + right, nil }
By implementing this type of check for each arithmetic operation, you can effectively detect and handle integer overflows in your Go code, ensuring the integrity of your computations and the robustness of your applications.
The above is the detailed content of How to Detect Signed Integer Overflow in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










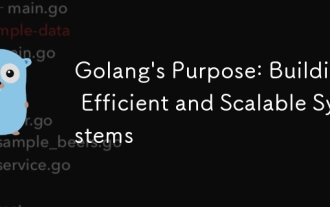
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
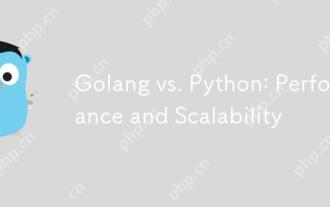
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
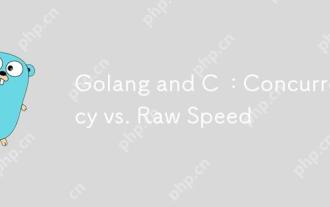
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
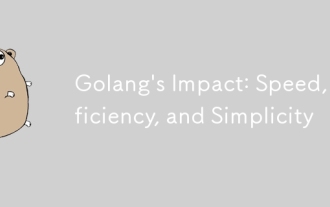
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
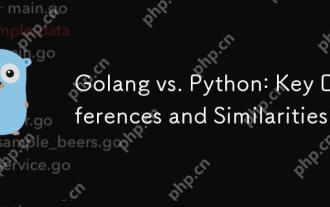
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
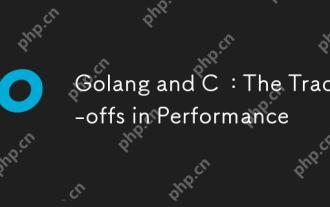
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
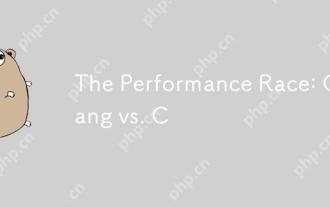
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
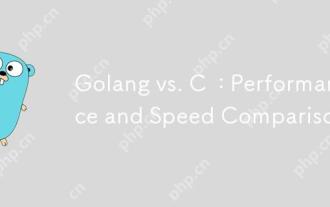
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
