How do I convert MySQL code to PDO statements?
How to convert MySQL code into PDO statement?
When working with databases in PHP, it's often necessary to convert MySQL code into PDO (PHP Data Objects) statements. PDO provides a consistent and secure way to interact with databases, regardless of the underlying driver. This guide will show you how to convert your MySQL code into PDO statements.
Make a connection
The first step is to make a connection to the database using PDO. Here's an example of how to do it:
$db_host = "127.0.0.1"; $db_name = "database_name"; $db_user = "username"; $db_pass = "password"; $pdo = new PDO("mysql:host=$db_host;dbname=$db_name", $db_user, $db_pass);
Updating your code
Once you have a PDO connection, you can start converting your MySQL code into PDO statements.
Prepared statements with mysqli and PDO
Prepared statements are a secure way to execute queries that contain user-supplied data. They prevent SQL injection by binding the data to the query before it is executed.
Here's an example of a prepared statement in MySQLi:
$sql = "SELECT * FROM users WHERE username = ? AND password = ?"; $stmt = $mysqli->prepare($sql); $stmt->bind_param("ss", $username, $password); $stmt->execute(); $result = $stmt->get_result();
Here's the same query in PDO:
$sql = "SELECT * FROM users WHERE username = ? AND password = ?"; $stmt = $pdo->prepare($sql); $stmt->bindParam(1, $username, PDO::PARAM_STR); $stmt->bindParam(2, $password, PDO::PARAM_STR); $stmt->execute(); $result = $stmt->fetchAll();
Updated code
Here's an example of how to convert the MySQL code from the question into PDO statements:
$id = $_SESSION['u_id'] ?? NULL; if ($id) { $sql = "SELECT email FROM users WHERE u_id = ?"; $stmt = $pdo->prepare($sql); $stmt->execute([$id]); $email = $stmt->fetchColumn(); } $email = $email ?? ""; $suggestions = selectAll($table); $optionOne = $_POST['optionOne'] ?? ""; $optionTwo = $_POST['optionTwo'] ?? ""; $newSuggestion = $_POST['new-suggestion'] ?? ""; if ($newSuggestion && $id && $email && $optionOne && $optionTwo) { $sql = "INSERT INTO suggestions (user_id, email, option_1, option_2) VALUES (?, ?, ?, ?)"; $stmt = $pdo->prepare($sql); $stmt->execute([$id, $email, $optionOne, $optionTwo]); } else { echo "All options must be entered"; }
Summary
Converting MySQL code into PDO statements is a relatively straightforward process. By following the steps outlined in this guide, you can easily migrate your code to PDO and take advantage of its benefits, such as improved security and performance.
The above is the detailed content of How do I convert MySQL code to PDO statements?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










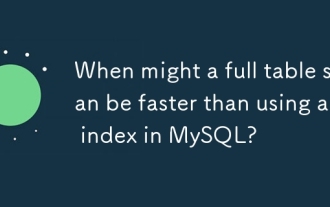
Full table scanning may be faster in MySQL than using indexes. Specific cases include: 1) the data volume is small; 2) when the query returns a large amount of data; 3) when the index column is not highly selective; 4) when the complex query. By analyzing query plans, optimizing indexes, avoiding over-index and regularly maintaining tables, you can make the best choices in practical applications.
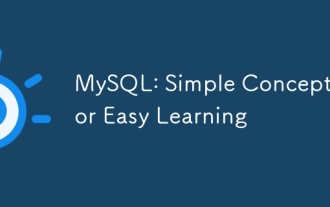
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
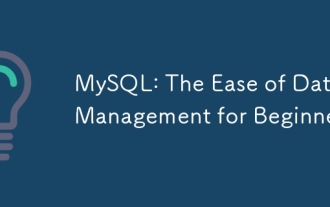
MySQL is suitable for beginners because it is simple to install, powerful and easy to manage data. 1. Simple installation and configuration, suitable for a variety of operating systems. 2. Support basic operations such as creating databases and tables, inserting, querying, updating and deleting data. 3. Provide advanced functions such as JOIN operations and subqueries. 4. Performance can be improved through indexing, query optimization and table partitioning. 5. Support backup, recovery and security measures to ensure data security and consistency.
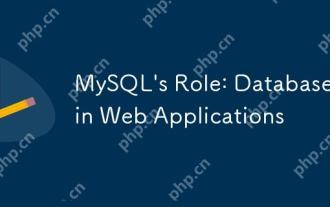
The main role of MySQL in web applications is to store and manage data. 1.MySQL efficiently processes user information, product catalogs, transaction records and other data. 2. Through SQL query, developers can extract information from the database to generate dynamic content. 3.MySQL works based on the client-server model to ensure acceptable query speed.
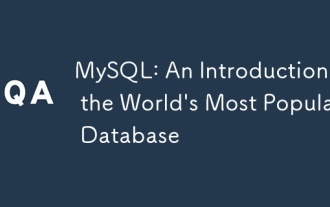
MySQL is an open source relational database management system, mainly used to store and retrieve data quickly and reliably. Its working principle includes client requests, query resolution, execution of queries and return results. Examples of usage include creating tables, inserting and querying data, and advanced features such as JOIN operations. Common errors involve SQL syntax, data types, and permissions, and optimization suggestions include the use of indexes, optimized queries, and partitioning of tables.
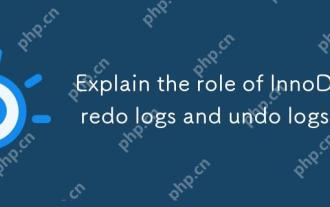
InnoDB uses redologs and undologs to ensure data consistency and reliability. 1.redologs record data page modification to ensure crash recovery and transaction persistence. 2.undologs records the original data value and supports transaction rollback and MVCC.
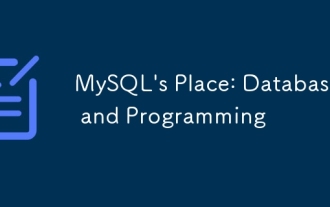
MySQL's position in databases and programming is very important. It is an open source relational database management system that is widely used in various application scenarios. 1) MySQL provides efficient data storage, organization and retrieval functions, supporting Web, mobile and enterprise-level systems. 2) It uses a client-server architecture, supports multiple storage engines and index optimization. 3) Basic usages include creating tables and inserting data, and advanced usages involve multi-table JOINs and complex queries. 4) Frequently asked questions such as SQL syntax errors and performance issues can be debugged through the EXPLAIN command and slow query log. 5) Performance optimization methods include rational use of indexes, optimized query and use of caches. Best practices include using transactions and PreparedStatemen
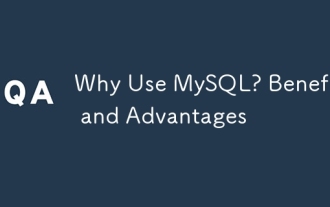
MySQL is chosen for its performance, reliability, ease of use, and community support. 1.MySQL provides efficient data storage and retrieval functions, supporting multiple data types and advanced query operations. 2. Adopt client-server architecture and multiple storage engines to support transaction and query optimization. 3. Easy to use, supports a variety of operating systems and programming languages. 4. Have strong community support and provide rich resources and solutions.
