


How Do You Prevent Reference Loss When Fetching Data Asynchronously with Firebase?
Delving into Firebase's Asynchronous Nature: Resolving Reference Loss
Firebase, coupled with AngularJS, enables efficient fetching of data, including lists of users. While accessing the user list with the once() function poses no difficulty, retrieving it beyond that function's scope proves elusive. This article explores the underlying causes and provides comprehensive solutions.
Understanding the Asynchronous Pitfall
Firebase's data retrieval operates asynchronously, rendering code execution non-linear. To illustrate this, consider the following code snippet:
this.getUsers = function() { var ref = firebase.database().ref('/users/'); ref.once('value').then(function(snapshot) { // User list processing console.log(users); // Output: All users }); console.log(userList); // Output: Undefined }
Upon execution, the expected user list output at the end remains elusive, despite the successful retrieval within the then() block. This arises because the code executes out of order:
- Log "before attaching listener"
- Attach listener to retrieve user data
- Log "after attaching listener"
- Upon listener completion, log "got value" and process the user list
- Log "undefined" since userList hasn't been assigned yet
Strategies for Capturing the User List
1. Utilize the Callback
A direct approach is to shift all user list-dependent code into the callback function. This restructures the logic from "load the list and then print" to "print whenever the list is loaded."
ref.once('value', function(snapshot) { // User list processing console.log(users); // Output: All users })
2. Leverage Promises and Callbacks
Promises offer a more elegant solution, allowing you to return a promise from your getUsers() function. This allows you to use the once() callback as before, but with an additional promise wrapping:
this.getUsers = function() { return ref.once('value').then(function(snapshot) { // User list processing return users; }) ... userService.getUsers().then(function(userList) { console.log(userList); })
3. Embrace Async/Await
With the use of promises, you can take advantage of the async/await syntax for a more synchronous-looking approach:
async function getAndLogUsers() { const userList = await userService.getUsers(); console.log(userList); }
However, it's important to note that the getUsers() function remains an asynchronous function, requiring the calling code to handle the Promise or Future accordingly.
By embracing these strategies, you can effectively harness Firebase's asynchronous capabilities and prevent the loss of reference beyond the once() function's scope.
The above is the detailed content of How Do You Prevent Reference Loss When Fetching Data Asynchronously with Firebase?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










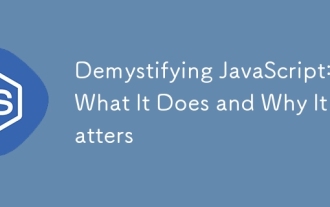
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
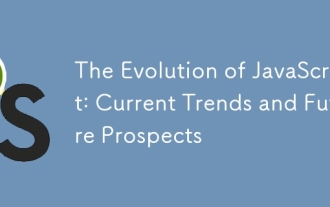
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
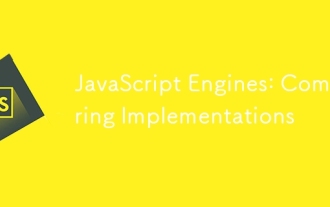
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
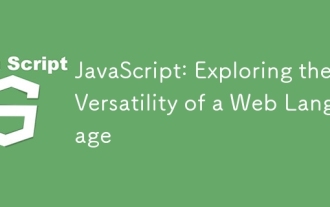
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
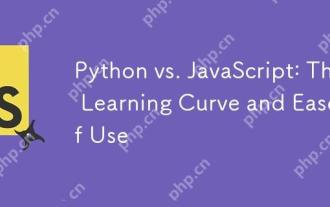
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
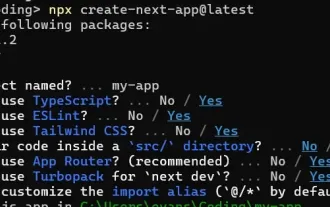
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
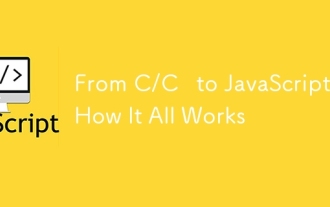
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
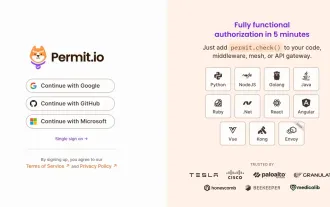
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
