


How do I Group Multidimensional Array Data by Multiple Columns and Aggregate Values in PHP?
Grouping Multidimensional Array Data by Multiple Columns and Aggregating Values
Problem Statement:
You have a multidimensional array that combines data from two separate database queries. The array consists of records with attributes that include "part," "address," "type," and "count." The goal is to group the array elements based on the "part" and "type" values and calculate the sum of the "count" values within each group.
Example Input:
<code class="php">$arr1 = [ ['part' => '1', 'address' => 'aaa', 'type' => '1', 'count' => 5], ['part' => '1', 'address' => 'bbb', 'type' => '1', 'count' => 5], ['part' => '1', 'address' => 'ccc', 'type' => '1', 'count' => 5], ['part' => '2', 'address' => 'aaa', 'type' => '1', 'count' => 5], ['part' => '2', 'address' => 'bbb', 'type' => '1', 'count' => 5], ['part' => '2', 'address' => 'ccc', 'type' => '2', 'count' => 5] ];</code>
Expected Output:
<code class="php">$arr2 = [ ['part' => '1', 'type' => '1', 'count' => 15], ['part' => '2', 'type' => '1', 'count' => 10], ['part' => '2', 'type' => '2', 'count' => 5] ];</code>
Solution:
To achieve the desired grouping and aggregation, we can utilize PHP's array manipulation functions. Consider the following function:
<code class="php">function groupByPartAndType($input) { $output = Array(); foreach($input as $value) { $output_element = & $output[$value['part'] . "_" . $value['type']]; $output_element['part'] = $value['part']; $output_element['type'] = $value['type']; !isset($output_element['count']) && $output_element['count'] = 0; $output_element['count'] += $value['count']; } return array_values($output); }</code>
By applying this function to the input array $arr1, we obtain the desired output $arr2. Each element in the output array represents a group of data points that share the same "part" and "type" values, along with the sum of the corresponding "count" values.
Alternative Approach:
If both database queries originate from the same database server, you could potentially use SQL's GROUP BY feature to perform the grouping and aggregation within the database itself, eliminating the need for post-processing in PHP.
The above is the detailed content of How do I Group Multidimensional Array Data by Multiple Columns and Aggregate Values in PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










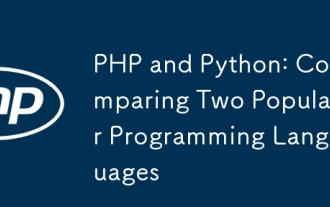
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
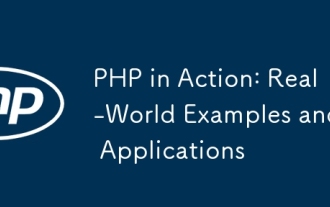
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
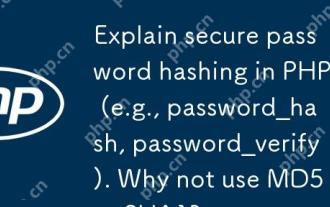
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
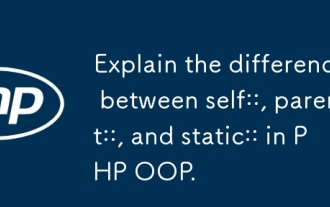
In PHPOOP, self:: refers to the current class, parent:: refers to the parent class, static:: is used for late static binding. 1.self:: is used for static method and constant calls, but does not support late static binding. 2.parent:: is used for subclasses to call parent class methods, and private methods cannot be accessed. 3.static:: supports late static binding, suitable for inheritance and polymorphism, but may affect the readability of the code.
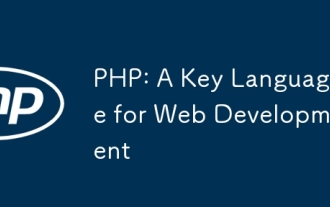
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
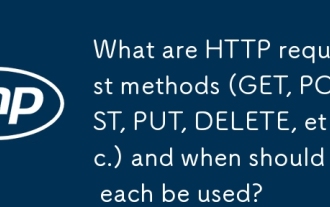
HTTP request methods include GET, POST, PUT and DELETE, which are used to obtain, submit, update and delete resources respectively. 1. The GET method is used to obtain resources and is suitable for read operations. 2. The POST method is used to submit data and is often used to create new resources. 3. The PUT method is used to update resources and is suitable for complete updates. 4. The DELETE method is used to delete resources and is suitable for deletion operations.
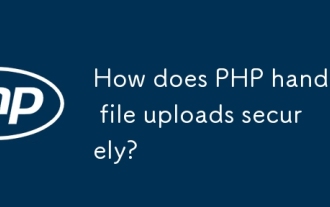
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
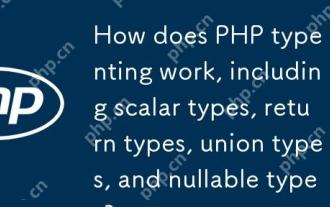
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
