


Accessor Methods vs. Dereferencing: Which is Better for Debugging Pointer Fields?
Accessor Methods vs. Dereferencing for Debugging
In this code snippet, you're printing the value of a struct field that is a pointer to another struct. The output is the memory address of the pointed-to struct, not the actual value.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
|
For debugging purposes, it's preferable to print the actual value of the field. There are two ways to achieve this: using accessor methods or dereferencing the pointer.
Accessor Methods
You can create getter methods for each pointer field to retrieve the actual value. For example:
1 2 3 4 5 6 |
|
Then, in your code, you can call the getter method to print the value:
1 |
|
This approach allows you to control the formatting of the output and provides a convenient way to access the field value without the need for dereferencing.
Dereferencing
If you prefer to dereference the pointer directly, you can use the following syntax:
1 |
|
However, this approach requires caution as dereferencing a nil pointer can lead to a runtime panic. It's recommended to use getter methods for safety unless you're certain the pointer is non-nil.
The above is the detailed content of Accessor Methods vs. Dereferencing: Which is Better for Debugging Pointer Fields?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
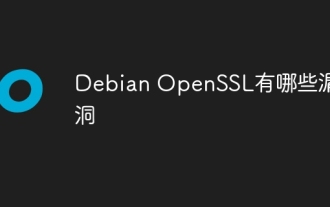
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
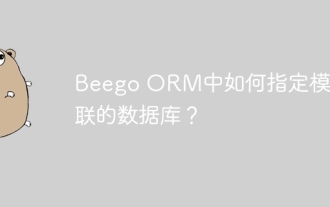
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
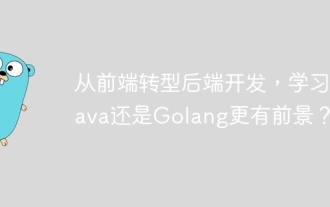
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
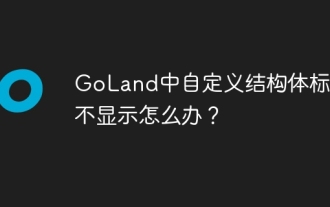
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
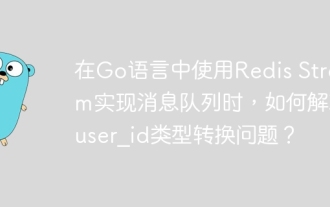
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
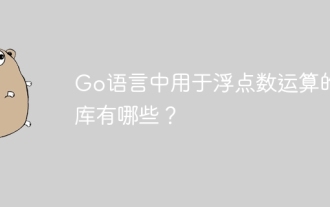
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
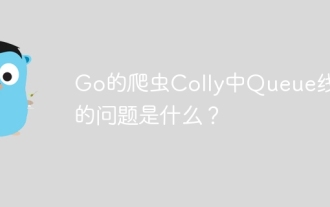
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
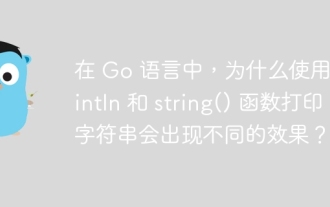
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
