


Does Pre-Compiling Regular Expressions with `re.compile()` Enhance Python Performance?
Performance Implications of Python's re.compile
In Python, the re module provides functionality for working with regular expressions. One question that often arises is whether there is a performance benefit in using the re.compile method to pre-compile regular expressions.
Using re.compile vs. Direct Matching
Consider the following two code snippets:
h = re.compile('hello') h.match('hello world')
re.match('hello', 'hello world')
The first snippet pre-compiles the regular expression 'hello' using re.compile() and then uses the compiled pattern to perform the match. The second snippet simply uses the re.match() function directly to perform the match.
Anecdotal Evidence and Code Analysis
Some users report that they have not observed any significant performance difference between using re.compile() and direct matching. This is supported by the fact that Python internally compiles regular expressions and caches them when they are used (including calls to re.match()).
The code analysis of the re module in Python 2.5 reveals that:
def match(pattern, string, flags=0): return _compile(pattern, flags).match(string) def _compile(*key): cachekey = (type(key[0]),) + key p = _cache.get(cachekey) if p is not None: return p # Actual compilation on cache miss if len(_cache) >= _MAXCACHE: _cache.clear() _cache[cachekey] = p return p
This shows that the primary difference between using re.compile() and direct matching is the timing of the compilation process. re.compile() forces the compilation to occur before the match is performed, while direct matching compiles the regular expression internally when the match function is called.
Conclusion
While pre-compiling regular expressions with re.compile() does not appear to offer significant performance gains, it can be useful for organizing and naming reusable patterns. However, it is important to be aware that Python caches compiled regular expressions internally, potentially reducing the perceived benefit of pre-compilation.
The above is the detailed content of Does Pre-Compiling Regular Expressions with `re.compile()` Enhance Python Performance?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










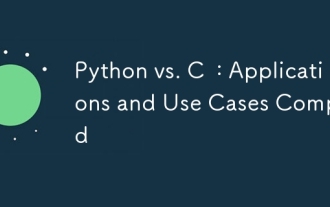
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
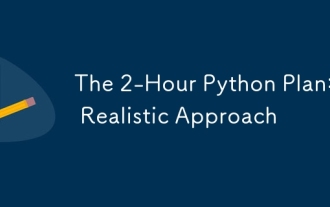
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
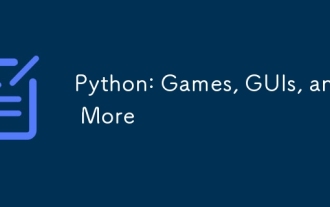
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
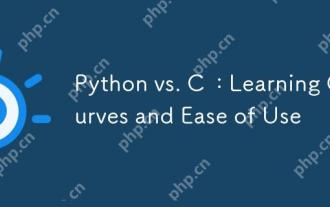
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
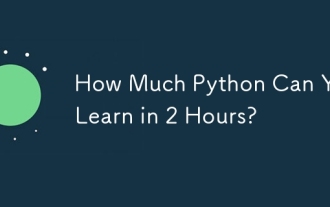
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
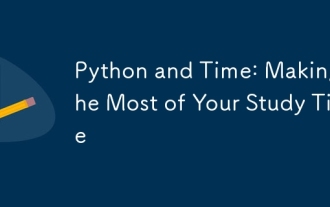
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
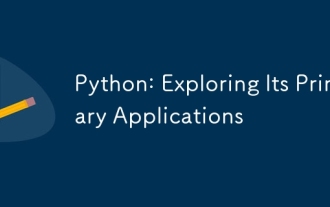
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
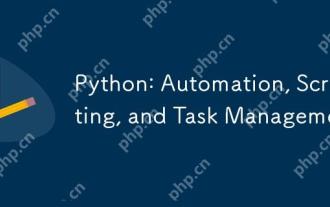
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
