How to Properly Handle Errors Returned by Defer in Go?
Error Handling and Deferrals
In Go, the defer statement is commonly used to execute a function or cleanup operation after the surrounding function returns. However, if the deferred function returns an error, it may be overlooked due to the typical practice of ignoring the error returned by defer, which can lead to unexpected system behavior.
Consider the following scenario:
OpenDbConnection(connectionString string, logSql bool) (*gorm.DB, error) { logger := zap.NewExample().Sugar() defer logger.Sync() }
In this example, the logger.Sync() method may return an error that is ignored, leaving potential issues unresolved.
Possible Strategies
- Use a named error variable: Initialize an error variable within the function scope and assign the error returned by the deferred function to it. This allows the error to be inspected and handled as needed.
- Callable argument-less deferred function: Enclose the deferred function within an anonymous function without arguments. This allows the deferred function to return an error, which can then be assigned to the named error variable.
- Return the error and handle it in the calling function: Return the error from the function and let the calling function handle it appropriately. This may be preferred if the error requires special handling or analysis.
Here is an example using the named error variable strategy:
func OpenDbConnection(connectionString string, logSql bool) (db *gorm.DB, err error) { logger := zap.NewExample().Sugar() defer func() { err = logger.Sync() }() // ... rest of function logic ... return db, err }
With this approach, the error can be checked and handled in the calling function:
db, err := OpenDbConnection(connectionString, logSql) if err != nil { // Handle the error }
The above is the detailed content of How to Properly Handle Errors Returned by Defer in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










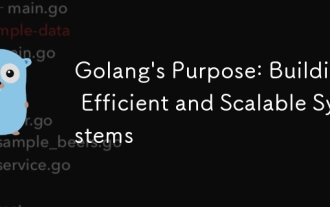
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
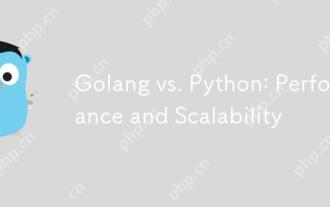
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
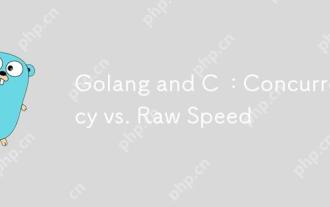
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
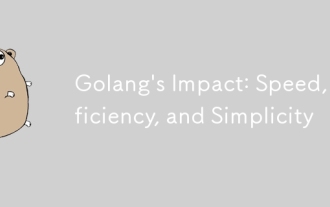
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
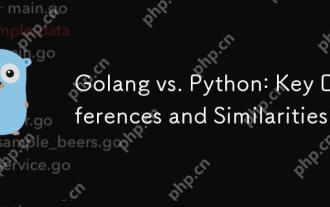
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
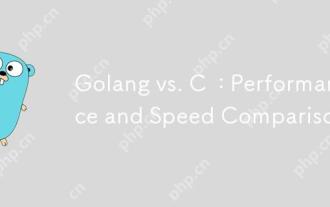
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
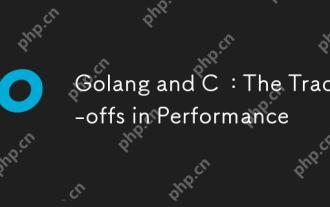
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
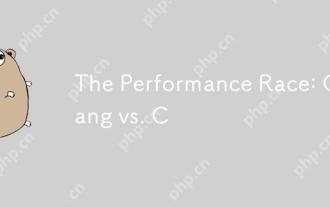
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
