How to Stream File Uploads to AWS S3 Using Go?
Stream File Upload to AWS S3 Using Go
Overview
Uploading large files directly to AWS S3 while minimizing memory and disk footprint is an essential task in cloud computing. This guide will demonstrate how to achieve this using the AWS SDK for Go.
Solution
To stream a file upload directly to S3, you can utilize the s3manager package. Here's a step-by-step solution:
-
Configure AWS Credentials and Session:
- Set your AWS access key and secret, or use the default credentials provider.
- Initialize an AWS session with the specified configuration.
-
Create an S3 Uploader:
- Initialize an S3 uploader with the session and optional configuration settings.
- You can configure parameters such as part size, concurrency, and max upload parts.
-
Open the File:
- Open the file you want to upload using the os.Open function.
-
Upload the File:
- Use the uploader.Upload method with the appropriate file information (bucket, key, and file pointer).
Example Code
The following code sample demonstrates how to stream large file upload to AWS S3 using s3manager:
package main import ( "fmt" "os" "github.com/aws/aws-sdk-go/aws/credentials" "github.com/aws/aws-sdk-go/aws" "github.com/aws/aws-sdk-go/aws/session" "github.com/aws/aws-sdk-go/service/s3/s3manager" ) const ( filename = "file_name.zip" myBucket = "myBucket" myKey = "file_name.zip" accessKey = "" accessSecret = "" ) func main() { var awsConfig *aws.Config if accessKey == "" || accessSecret == "" { //load default credentials awsConfig = &aws.Config{ Region: aws.String("us-west-2"), } } else { awsConfig = &aws.Config{ Region: aws.String("us-west-2"), Credentials: credentials.NewStaticCredentials(accessKey, accessSecret, ""), } } // The session the S3 Uploader will use sess := session.Must(session.NewSession(awsConfig)) // Create an uploader with the session and default options //uploader := s3manager.NewUploader(sess) // Create an uploader with the session and custom options uploader := s3manager.NewUploader(sess, func(u *s3manager.Uploader) { u.PartSize = 5 * 1024 * 1024 // The minimum/default allowed part size is 5MB u.Concurrency = 2 // default is 5 }) //open the file f, err := os.Open(filename) if err != nil { fmt.Printf("failed to open file %q, %v", filename, err) return } //defer f.Close() // Upload the file to S3. result, err := uploader.Upload(&s3manager.UploadInput{ Bucket: aws.String(myBucket), Key: aws.String(myKey), Body: f, }) //in case it fails to upload if err != nil { fmt.Printf("failed to upload file, %v", err) return } fmt.Printf("file uploaded to, %s\n", result.Location) }
By following these steps, you can efficiently upload large multipart/form-data files directly to AWS S3 with minimal memory utilization.
The above is the detailed content of How to Stream File Uploads to AWS S3 Using Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










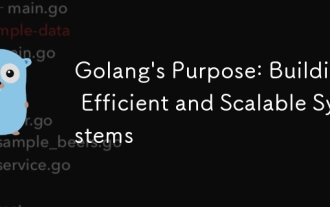
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
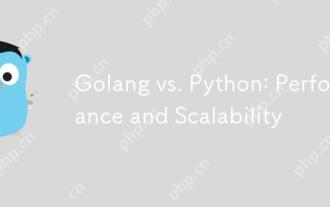
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
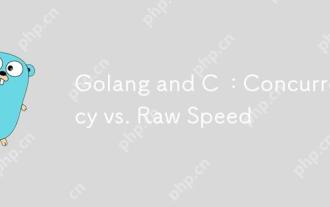
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
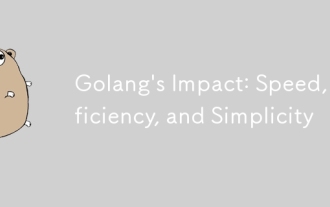
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
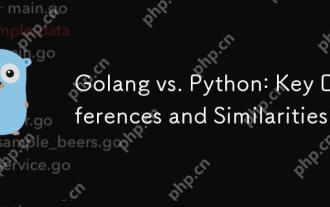
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
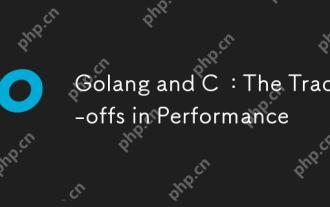
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
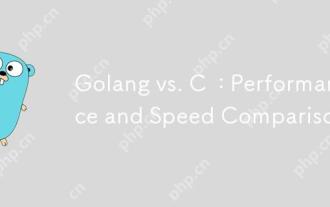
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
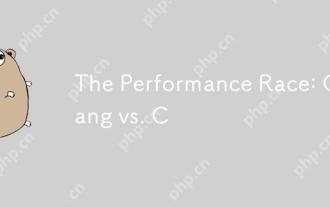
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
