What Causes an InputMismatchException in Java and How to Fix It?
InputMismatchException in Java Code: Troubleshooting Input
When using the Scanner class in Java, unexpected exceptions can arise during input processing. One common error is the InputMismatchException, which occurs when the input entered doesn't match the expected data type.
Consider this code snippet:
1 2 3 4 5 6 7 8 9 10 |
|
and
1 2 3 4 5 6 7 8 9 10 |
|
When testing this code, you encounter an InputMismatchException due to an improper data type being entered. The issue arises when attempting to enter a double value using a dot (.) as the decimal separator.
Solution:
To resolve this problem, use a comma (,), not a dot, to separate the fractional part of the number. For example, instead of entering 1.2, input 1,2. The comma is the default decimal separator for double data types in Java.
By making this simple modification, you can ensure that the code accepts double values correctly and avoids the InputMismatchException.
The above is the detailed content of What Causes an InputMismatchException in Java and How to Fix It?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










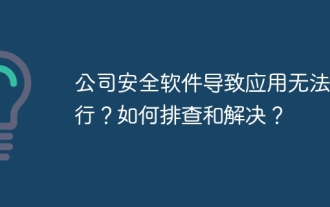
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
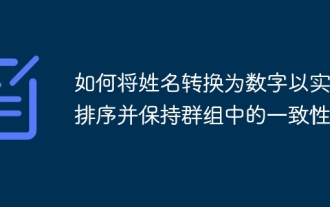
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
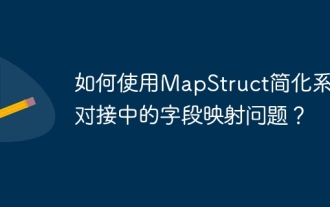
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
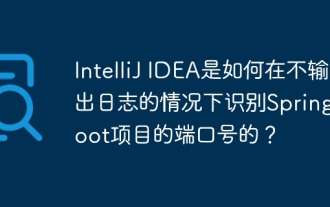
Start Spring using IntelliJIDEAUltimate version...
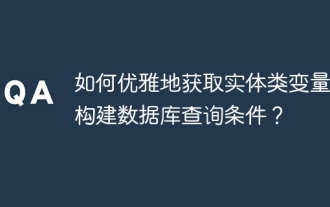
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
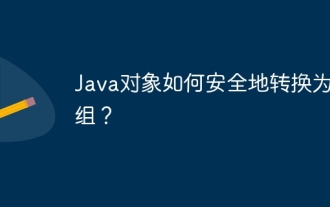
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
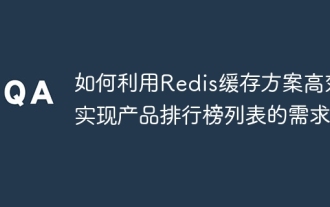
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
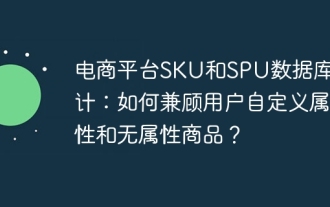
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
