How to Concatenate Strings in a Single Line in C ?
Nov 08, 2024 am 02:25 AMConcatenating Strings in a Single Line in C
When working with strings in programming, it can be convenient to concatenate multiple strings together. In some languages like C#, this can be done concisely on a single line. However, if you're using C , you might be wondering how to achieve similar functionality.
In C , there isn't a built-in way to concatenate multiple strings with the ' ' operator on one line like in C#. However, using a stringstream enables you to do so easily.
Utilizing Stringstream
To concatenate multiple strings in a single line in C , you can use std::stringstream. This class provides a stream interface to strings, allowing you to manipulate them using streaming operations. Here's how you can concatenate strings using a stringstream:
#include <sstream> #include <string> std::stringstream ss; ss << "Hello, world, " << myInt << niceToSeeYouString; std::string s = ss.str();
In this code:
std::stringstream ss; creates a stringstream object.
ss << "Hello, world, " << myInt << niceToSeeYouString;: We concatenate strings and variables using the insertion operator (<<) into the stringstream.
std::string s = ss.str();: Finally, we extract the concatenated string from the stringstream and store it in the std::string variable s.
The above is the detailed content of How to Concatenate Strings in a Single Line in C ?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
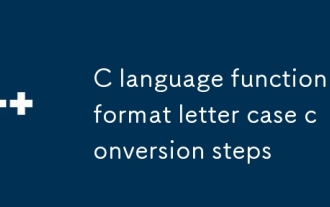
C language function format letter case conversion steps
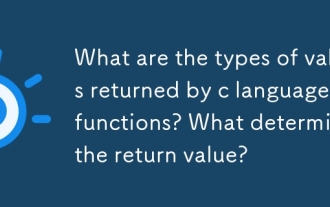
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the
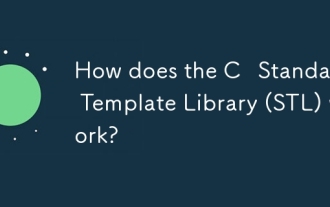
How does the C Standard Template Library (STL) work?

Where is the return value of the c language function stored in memory?

What is the minimum common multiple of the maximum common divisor of a c language function?
