


How can I efficiently process gigantic CSV files in Python 2.7 without running into memory issues?
Reading Gigantic CSV Files: Optimizing Memory and Speed
When attempting to process massive CSV files with millions of rows and hundreds of columns, traditional approaches using iterators can lead to memory-related issues. This article explores optimized techniques for handling large-scale CSV data in Python 2.7.
Memory Optimization:
The crux of the memory problem lies in constructing in-memory lists to store large datasets. To mitigate this issue, Python offers the yield keyword, which converts functions into generator functions. These functions pause execution after each yield statement, allowing incremental processing of data as it's encountered.
By employing generator functions, you can process data row by row, eliminating the need to store entire files in memory. The following code demonstrates this approach:
import csv def getstuff(filename, criterion): with open(filename, "rb") as csvfile: datareader = csv.reader(csvfile) yield next(datareader) # yield header row count = 0 for row in datareader: if row[3] == criterion: yield row count += 1 elif count: # stop processing when a consecutive series of non-matching rows is encountered return
Speed Enhancements:
Additionally, you can leverage Python's dropwhile and takewhile functions to further improve processing speed. These functions can filter data efficiently, enabling you to quickly locate the rows of interest. Here's how:
from itertools import dropwhile, takewhile def getstuff(filename, criterion): with open(filename, "rb") as csvfile: datareader = csv.reader(csvfile) yield next(datareader) # yield header row yield from takewhile( # yield matching rows lambda r: r[3] == criterion, dropwhile( # skip non-matching rows lambda r: r[3] != criterion, datareader)) return
Simplified Looped Processing:
By combining generator functions, you can greatly simplify the process of looping through your dataset. Here's the optimized code for getstuff and getdata:
def getdata(filename, criteria): for criterion in criteria: for row in getstuff(filename, criterion): yield row
Now, you can directly iterate over the getdata generator, which produces a stream of rows row by row, freeing up valuable memory resources.
Remember, the goal is to minimize in-memory data storage while simultaneously maximizing processing efficiency. By applying these optimization techniques, you can effectively handle gigantic CSV files without encountering memory roadblocks.
The above is the detailed content of How can I efficiently process gigantic CSV files in Python 2.7 without running into memory issues?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










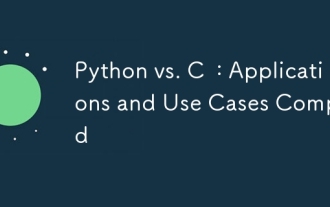
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
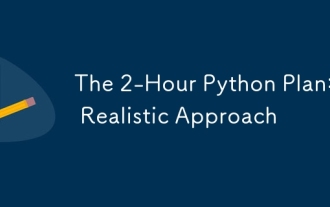
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
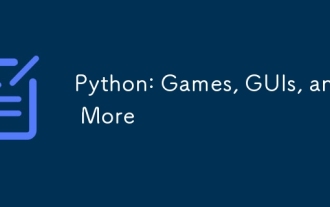
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
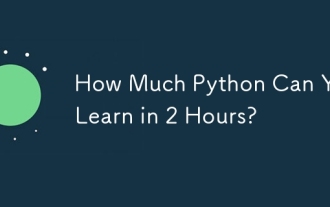
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
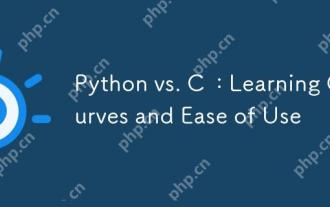
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
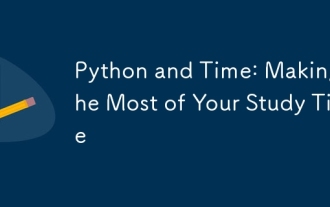
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
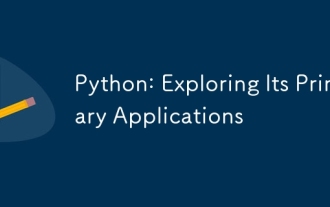
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
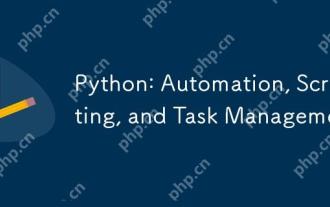
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
