How Does Short Circuit Evaluation Work in Go?
Short Circuit Evaluation in Go
In computer programming, short circuit evaluation is an optimization technique that improves the performance of conditional statements by skipping the evaluation of subsequent conditions once one condition is met. This behavior is intended to avoid unnecessary computation, especially when one condition implicitly implies the outcome of the others.
Go's Short Circuit Evaluation
Go follows the principle of short circuit evaluation. In other words, in an if statement, Go only evaluates subsequent conditions if the preceding ones are not met. This applies to both the if-else statement and the if statement without an else clause.
Performance Comparison
Let's analyze the two code snippets provided in the question:
if !isValidQueryParams(&queries) || r == nil || len(queries) == 0 { return "", fmt.Errorf("invalid querystring") }
if r == nil || len(queries) == 0 || !isValidQueryParams(&queries) { return "", fmt.Errorf("invalid querystring") }
In both cases, if r == nil or len(queries) == 0, the isValidQueryParams function will not be called because the overall expression is already false. Therefore, the performance optimizations may not be significant in this particular context.
Example
To demonstrate short circuit evaluation in action, consider the following code:
package main import "fmt" func main() { for i := 0; i < 10; i++ { if testFunc(1) || testFunc(2) { // do nothing } } } func testFunc(i int) bool { fmt.Printf("function %d called\n", i) return true }
Running this code will print:
function 1 called function 1 called function 1 called function 1 called function 1 called function 1 called function 1 called function 1 called function 1 called function 1 called
As you can see, the testFunc function with argument 2 is never called because the first condition (testFunc(1)) always evaluates to true. This illustrates how short circuit evaluation prevents unnecessary function calls.
The above is the detailed content of How Does Short Circuit Evaluation Work in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










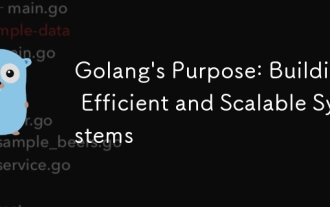
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
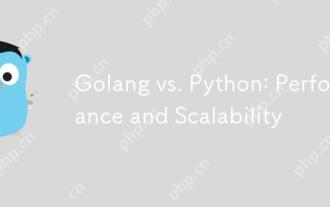
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
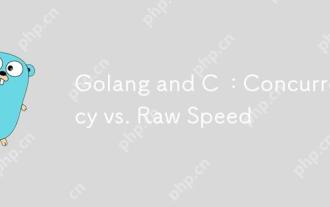
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
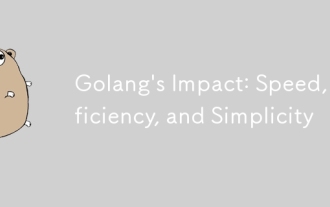
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
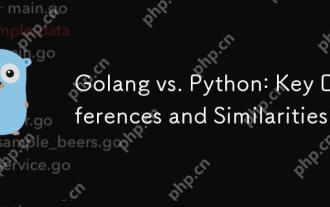
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
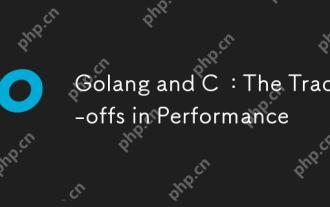
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
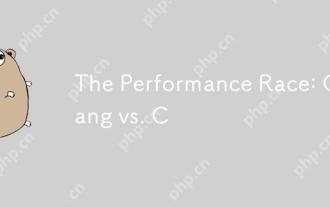
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
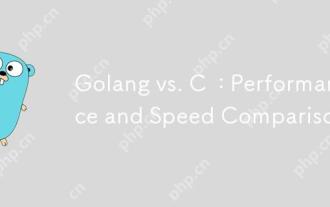
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
