


How can I automatically request administrator privileges for my Go application in Windows?
Requesting Administrator Privileges in Windows with Go
This article explores how to eliminate the need for manually selecting "Run as administrator" when launching an application, enabling it to prompt for admin permissions automatically.
Issue Description:
The question stems from the inability to write to a Windows system folder (C:Windows) without elevated privileges. When running the provided code, the application fails to write to the test.txt file with an "Access is denied" error.
Solution:
To address this issue, a method involving self-relaunching the application with elevated privileges is presented. This technique utilizes the following approach:
1. Checking for Admin Status:
The amAdmin() function checks if the application is running as an administrator by attempting to open the .PHYSICALDRIVE0 device. If access is granted, the function returns true; otherwise, it returns false.
2. Relaunching with Elevation:
If amAdmin() returns false, the runMeElevated() function is executed. This function uses the Windows ShellExecute API to relaunch the application with elevated privileges. It specifies the "runas" verb, which prompts the user for administrator permission.
3. Main Function Execution:
The main function first checks if the application has administrator privileges. If not, it invokes the runMeElevated() function to relaunch itself as administrator. If the application is already running with elevated privileges, it proceeds to execute the intended code.
Code Sample:
package main import ( "fmt" "golang.org/x/sys/windows" "os" "syscall" "time" ) func main() { // If not elevated, relaunch by shellexecute with runas verb set if !amAdmin() { runMeElevated() } time.Sleep(10 * time.Second) } func runMeElevated() { verb := "runas" exe, _ := os.Executable() cwd, _ := os.Getwd() args := strings.Join(os.Args[1:], " ") verbPtr, _ := syscall.UTF16PtrFromString(verb) exePtr, _ := syscall.UTF16PtrFromString(exe) cwdPtr, _ := syscall.UTF16PtrFromString(cwd) argPtr, _ := syscall.UTF16PtrFromString(args) var showCmd int32 = 1 //SW_NORMAL err := windows.ShellExecute(0, verbPtr, exePtr, argPtr, cwdPtr, showCmd) if err != nil { fmt.Println(err) } } func amAdmin() bool { _, err := os.Open("\\.\PHYSICALDRIVE0") if err != nil { fmt.Println("admin no") return false } fmt.Println("admin yes") return true }
By implementing this method, the application will automatically prompt for administrator permission when launched normally, eliminating the need for manual elevation via the right-click menu.
The above is the detailed content of How can I automatically request administrator privileges for my Go application in Windows?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










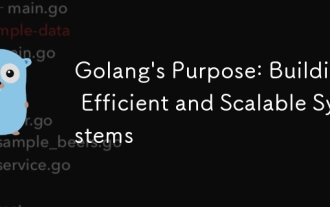
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
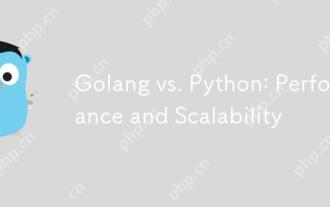
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
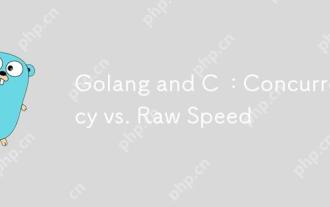
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
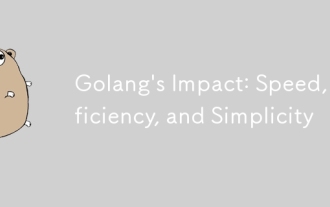
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
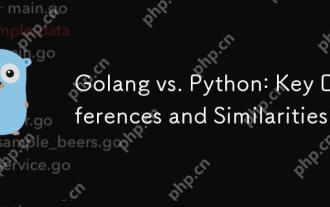
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
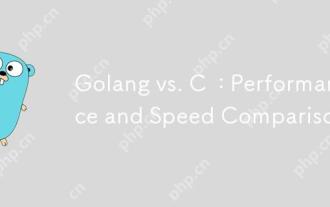
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
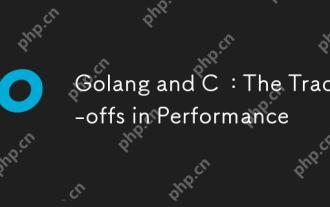
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
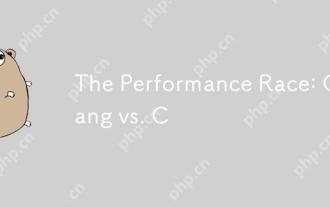
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
