


How to Recursively Populate Hierarchical Categories in MySQL with a Single Query?
Populating Recursive Categories with a Single MySQL Query
Organizing a website's content into hierarchical categories poses a challenge when it comes to efficiently retrieving those categories for display. This article delves into the most effective approach to recursively retrieve category data using PHP and MySQL.
Recursive Structure: A Hierarchical Tree
Imagine a website with articles and sections organized in a tree-like structure. Each section may have a parent section, potentially leading to multiple levels of nesting. For instance:
-
Subject 1
-
Subject 2
- Subject 3
-
Subject 4
- Subject 5
-
Subject 6
- Subject 7
-
- Subject 8
- Subject 9
MySQL Query: Fetching Parent-Child Relationships
To retrieve this data recursively, we need to fetch the parent-child relationships from the MySQL database. The query below accomplishes this task:
SELECT category_id, name, parent FROM categories ORDER BY parent
PHP Script: Constructing the Tree Structure
Once the data is fetched, we can build the tree structure in PHP to handle complex nesting scenarios. Here's an example script:
$nodeList = array(); // Associative array to store category nodes $tree = array(); // Array to hold the root nodes // Populate the $nodeList array with category data $query = mysql_query("SELECT category_id, name, parent FROM categories ORDER BY parent"); while ($row = mysql_fetch_assoc($query)) { $nodeList[$row['category_id']] = array_merge($row, array('children' => array())); } mysql_free_result($query); // Populate the $tree array with root nodes (those without a parent) foreach ($nodeList as $nodeId => &$node) { if (!$node['parent'] || !array_key_exists($node['parent'], $nodeList)) { $tree[] = &$node; } else { // If the node has a parent, add it as a child of that parent $nodeList[$node['parent']]['children'][] = &$node; } } // Clean up the variables unset($node); unset($nodeList); // The $tree array now contains the hierarchical tree structure
Performance Considerations
This PHP-based approach is particularly efficient, even for large trees. It avoids the overhead of making multiple recursive MySQL queries, which can significantly slow down performance.
Conclusion
This efficient PHP and MySQL solution allows you to recursively retrieve category data without sacrificing performance. By leveraging a clever reference-based approach, we can build complex hierarchical structures without the need for complex database queries.
The above is the detailed content of How to Recursively Populate Hierarchical Categories in MySQL with a Single Query?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


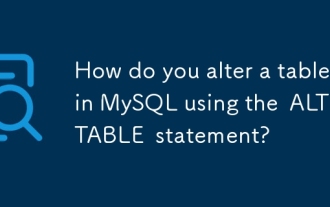
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
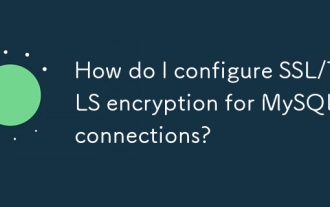
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
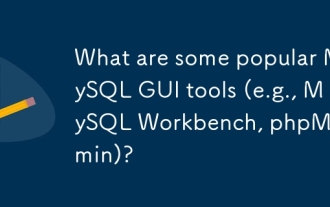
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]
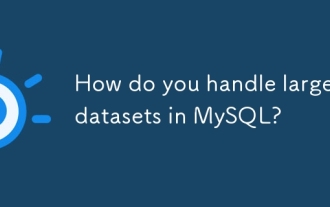
Article discusses strategies for handling large datasets in MySQL, including partitioning, sharding, indexing, and query optimization.
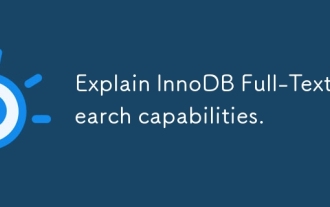
InnoDB's full-text search capabilities are very powerful, which can significantly improve database query efficiency and ability to process large amounts of text data. 1) InnoDB implements full-text search through inverted indexing, supporting basic and advanced search queries. 2) Use MATCH and AGAINST keywords to search, support Boolean mode and phrase search. 3) Optimization methods include using word segmentation technology, periodic rebuilding of indexes and adjusting cache size to improve performance and accuracy.
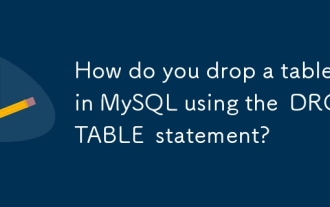
The article discusses dropping tables in MySQL using the DROP TABLE statement, emphasizing precautions and risks. It highlights that the action is irreversible without backups, detailing recovery methods and potential production environment hazards.
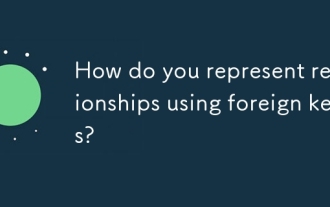
Article discusses using foreign keys to represent relationships in databases, focusing on best practices, data integrity, and common pitfalls to avoid.
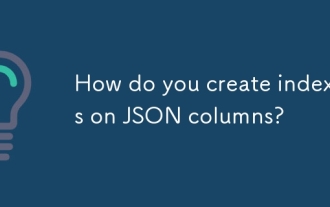
The article discusses creating indexes on JSON columns in various databases like PostgreSQL, MySQL, and MongoDB to enhance query performance. It explains the syntax and benefits of indexing specific JSON paths, and lists supported database systems.
