


Does a Failed os.FindProcess Call in Go Necessarily Mean a Process Has Terminated?
Determining Process Existence in Go
In Go, the os.FindProcess function can be used to retrieve a process by its PID. However, if this function returns an error, does it necessarily indicate that the process has terminated?
Checking for Process Existence
According to the man page for kill(2) in Unix, sending a signal of 0 to a process does not actually send a signal but checks if the process is alive. This approach can be adapted in Go to determine the existence of a process.
Go Implementation
The following Go code demonstrates this technique:
package main import ( "fmt" "log" "os" "strconv" "syscall" ) func main() { for _, p := range os.Args[1:] { pid, err := strconv.ParseInt(p, 10, 64) if err != nil { log.Fatal(err) } process, err := os.FindProcess(int(pid)) if err != nil { fmt.Printf("Failed to find process: %s\n", err) } else { err := process.Signal(syscall.Signal(0)) fmt.Printf("process.Signal on pid %d returned: %v\n", pid, err) } } }
Sample Output
When run, this code will display the status of multiple processes:
$ ./kill 1 $$ 123 process.Signal on pid 1 returned: operation not permitted process.Signal on pid 12606 returned: <nil> process.Signal on pid 123 returned: no such process
In this example, process 1 returns an error because it is not owned by the current user. Process 12606 returns nil because it is alive and owned by the user. Process 123 returns an error because it no longer exists.
The above is the detailed content of Does a Failed os.FindProcess Call in Go Necessarily Mean a Process Has Terminated?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










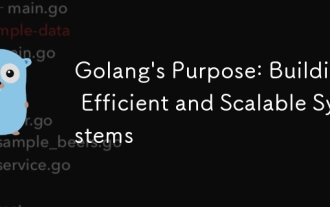
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
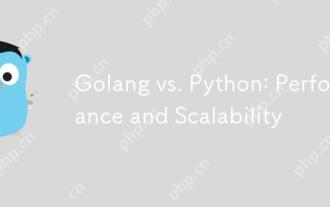
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
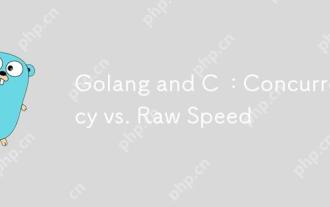
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
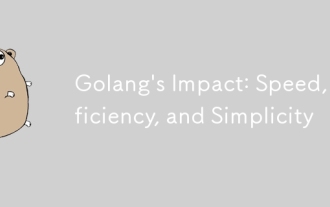
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
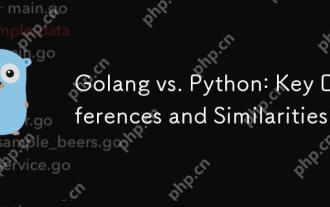
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
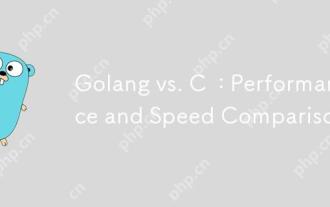
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
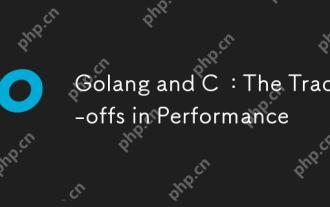
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
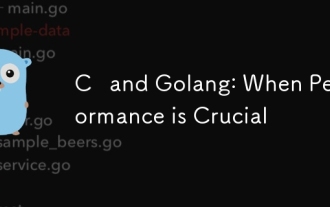
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
