


How to Convert a String Representation of a List into a List Object in Python?
Converting a String Representation of a List into a List Object
When dealing with data, it's common to encounter string representations of lists. Consider the following example:
fruits = "['apple', 'orange', 'banana']"
Our goal is to convert this string into an actual list object. This conversion is necessary for accessing and manipulating list elements effectively.
To achieve this, we can leverage the ast module in Python, which provides methods for evaluating Python expressions and literals. In this case, we'll employ the ast.literal_eval() function.
import ast fruits = "['apple', 'orange', 'banana']" fruits = ast.literal_eval(fruits)
By using ast.literal_eval(), we securely evaluate the string as a Python expression, resulting in a list object. We can verify this transformation by printing the list:
print(fruits) # Output: ['apple', 'orange', 'banana']
Furthermore, we can access list elements using square brackets:
print(fruits[1]) # Output: 'orange'
It's worth noting that ast.literal_eval() is safe for evaluating strings containing Python expressions. This safety measure is particularly important when working with untrusted sources, as it prevents potential security vulnerabilities.
The above is the detailed content of How to Convert a String Representation of a List into a List Object in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
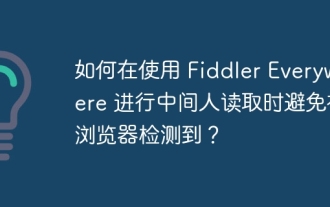
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
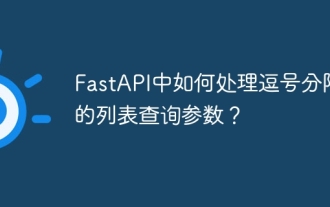
Fastapi ...
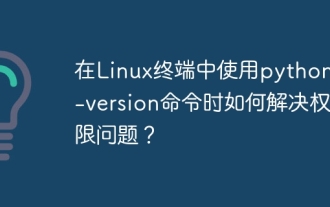
Using python in Linux terminal...
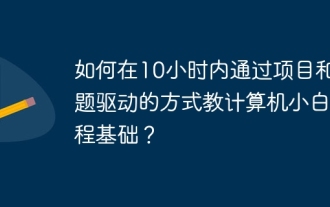
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
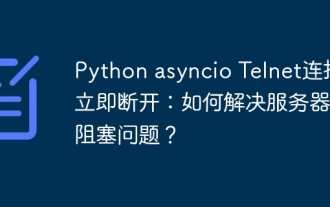
About Pythonasyncio...
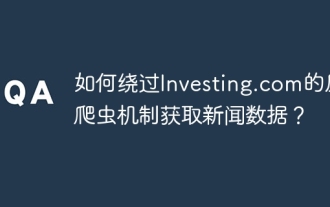
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
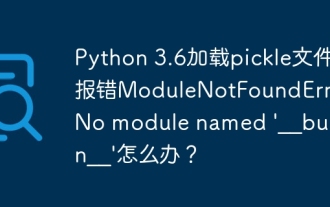
Loading pickle file in Python 3.6 environment error: ModuleNotFoundError:Nomodulenamed...
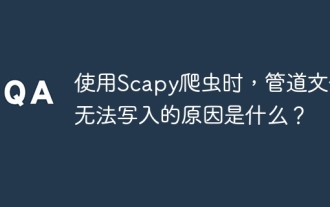
Discussion on the reasons why pipeline files cannot be written when using Scapy crawlers When learning and using Scapy crawlers for persistent data storage, you may encounter pipeline files...
