


How to Implement Equality Comparison in Python Classes Elegantly?
Elegant Approaches for Equality Comparison in Python Classes
The Challenge
Defining custom classes in Python often requires implementing equality comparison through the use of special methods, eq and ne__. A common approach involves comparing the __dict attributes of the instances.
A Closer Look at the dict Comparison Method
Comparing __dict__s provides an easy way to check for equality:
def __eq__(self, other): if isinstance(other, self.__class__): return self.__dict__ == other.__dict__ else: return False
While this approach is convenient, it may have drawbacks:
- Inherit from dict: Comparing dict essentially treats your class as an extended dictionary.
- Hash conflict: Objects with identical dict may have different hashes, affecting set and dictionary behavior.
Alternative Approaches
There are more elegant alternatives to using dict comparison:
1. Define __slots__:
Declare slots in your class to limit instance attributes to specific ones:
class Foo: __slots__ = ['item'] def __init__(self, item): self.item = item
This ensures that comparison is efficient and prevents adding arbitrary attributes to instances.
2. Use namedtuple:
Leverage Python's namedtuples to quickly define classes with predefined attributes:
from collections import namedtuple Foo = namedtuple('Foo', ['item'])
namedtuples support equality comparison out of the box.
3. Define hash and __eq__:
Override hash to return a hash based on the important class attributes, ensuring unique hashes for equal objects. Then, implement eq to compare objects based on their attributes, not their hashes:
class Foo: def __init__(self, item): self.item = item def __hash__(self): return hash(self.item) def __eq__(self, other): return self.item == other.item
4. Use metaclasses:
Metaclasses allow you to dynamically create classes with custom behavior. You can create a metaclass that automagically defines eq and ne methods based on the class attributes:
class MyMeta(type): def __new__(cls, name, bases, dct): attributes = tuple(dct.keys()) def __eq__(self, other): return all(getattr(self, attr) == getattr(other, attr) for attr in attributes) dct['__eq__'] = __eq__ return super().__new__(cls, name, bases, dct)
5. Inherit from a Custom Base Class:
Create a base class with eq and hash already defined for the desired behavior. Other classes can inherit from this base class to benefit from its equality comparison functionality.
Conclusion
While comparing __dict__s can be a simple solution, there are more elegant and efficient approaches available for implementing equality comparison in Python classes. The choice of method depends on the specific requirements of your application.
The above is the detailed content of How to Implement Equality Comparison in Python Classes Elegantly?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










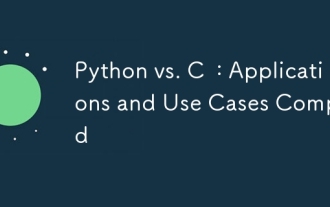
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
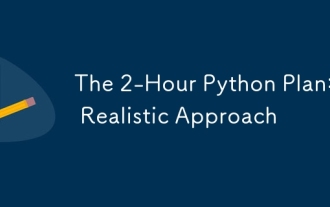
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
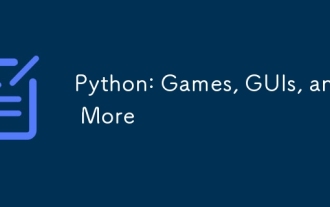
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
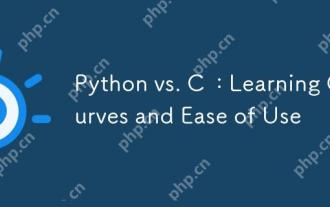
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
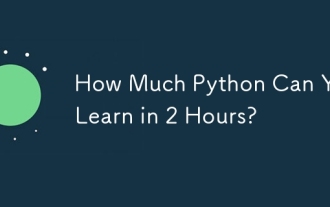
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
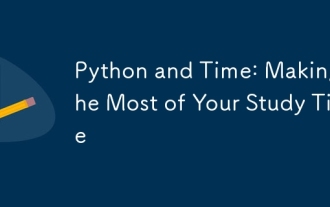
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
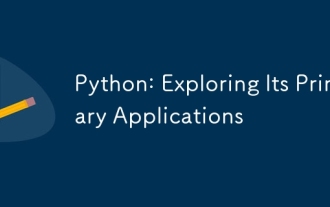
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
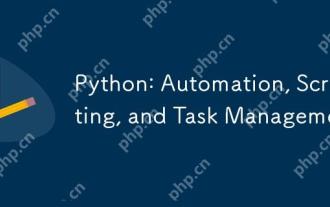
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
