


How can I manage and define global variables in a webpack project efficiently?
Define Global Variables with Webpack
Defining global variables in a webpack project allows you to access them from any module without the need for explicit imports. Here are several approaches to achieve this:
1. Module-Based Global Variables
Place your variables in a module, such as globals.js. Export an object containing your global variables and import it in subsequent modules. This ensures that the instance remains global and retains changes across modules.
Example:
// globals.js export default { FOO: 'bar' } // somefile.js import CONFIG from './globals.js' console.log(`FOO: ${CONFIG.FOO}`)
2. ProvidePlugin
Webpack's ProvidePlugin makes a module available as a global variable only in modules where it's used. This is useful for importing commonly used modules without explicit imports.
Example:
// webpack.config.js module.exports = { plugins: [ new webpack.ProvidePlugin({ 'utils': 'utils' }) ] } // any-file.js utils.sayHello() // Call the global function from 'utils.js'
3. DefinePlugin
For string-based constants, use Webpack's DefinePlugin to define global variables. These variables are available as string literals.
Example:
// webpack.config.js module.exports = { plugins: [ new webpack.DefinePlugin({ VERSION: JSON.stringify("5fa3b9") }) ] } // any-file.js console.log(`Running App version ${VERSION}`)
4. Window/Global Objects
In browser environments, define global variables through the window object. In Node.js environments, use the global object.
Example:
// Browser environment window.myVar = 'test' // Node.js environment global.myVar = 'test'
5. dotenv Package (Server Side)
For server-side projects, use the dotenv package to load environment variables from a local configuration file into the process.env object.
Example:
// Require dotenv require('dotenv').config() // Use environment variables var dbHost = process.env.DB_HOST
Notes:
- Use Webpack's Externals to exclude modules from the built bundle and make them globally available from external sources.
- The approach you choose depends on your specific requirements and the type of project you're working with. Consider factors such as module reuse, performance, and maintainability.
The above is the detailed content of How can I manage and define global variables in a webpack project efficiently?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
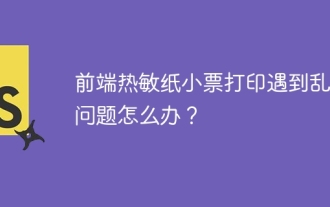
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
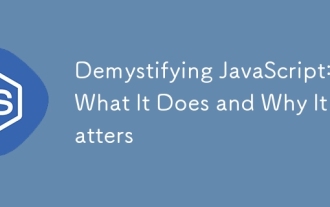
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
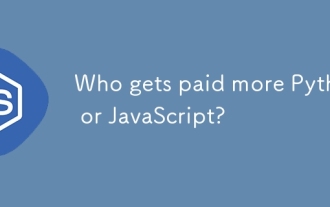
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
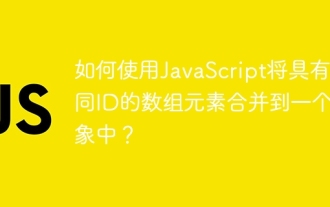
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
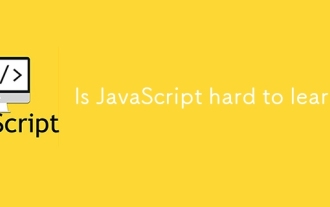
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
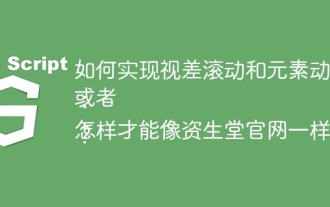
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
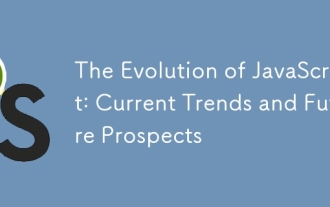
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
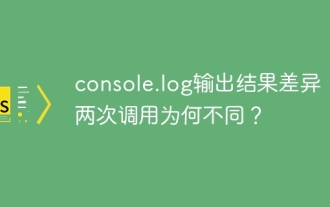
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
