


How Can I Insert and Retrieve PostGIS Geometries with Gorm in Golang?
Inserting and Selecting PostGIS Geometry with Gorm: A Comprehensive Guide
Using Golang, you can effortlessly insert and retrieve geometric types via Gorm, a popular ORM. This guide will walk you through the process, utilizing the orb library for defining and encoding/decoding geometries.
Orb and Well-Known Binary (WKB) Format
Orb offers Scan() and Value() methods, enabling Gorm's Insert() and Scan() functions to work beyond primitives. Orb requires geometries in the WKB format. Hence, we use the PostGIS functions ST_AsBinary() and ST_GeomFromWKB() during querying and inserting, respectively.
Challenges with Gorm
Gorm automatically handles value insertion and data scanning, making it challenging to apply our custom functions. Direct insertion of binary data fails, while querying yields results in hex format.
Potential Solutions
a. Views: Creating views that automatically apply the required functions can facilitate querying, but not insertion.
b. Triggers or Rules: Automating function calls on incoming/outgoing data via triggers or rules could offer a solution but is not universally applicable.
c. Custom Data Model Scanning: Scanning the entire data model and programmatically generating queries is a possible approach but is suboptimal.
Solution with GeoJSON Encoding
An effective alternative to Orb is to employ the geojson encoding library. This approach eliminates the need for manual byte manipulation:
Code:
<code class="go">import "github.com/twpayne/go-geom/encoding/geojson" type EWKBGeomPoint geom.Point func (g *EWKBGeomPoint) Scan(input interface{}) error { gt, err := ewkb.Unmarshal(input.([]byte)) if err != nil { return err } g = gt.(*EWKBGeomPoint) return nil } func (g EWKBGeomPoint) Value() (driver.Value, error) { b := geom.Point(g) bp := &b ewkbPt := ewkb.Point{Point: bp.SetSRID(4326)} return ewkbPt.Value() } type Track struct { gorm.Model GeometryPoint EWKBGeomPoint `gorm:"column:geom"` }</code>
Table Setup Customization:
<code class="go">err := db.Exec(`CREATE TABLE IF NOT EXISTS tracks ( id SERIAL PRIMARY KEY, geom geometry(POINT, 4326) NOT NULL );`).Error if err != nil { return err } err = gormigrate.New(db, gormigrate.DefaultOptions, []*gormigrate.Migration{ { ID: "init", Migrate: func(tx *gorm.DB) error { return tx.CreateTable( Tables..., ).Error }, }, { ID: "tracks_except_geom", Migrate: func(tx *gorm.DB) error { return db.AutoMigrate(Track{}).Error }, }, }).Migrate()</code>
With these modifications, you can seamlessly insert and extract geometric types using Gorm, empowering your Go applications with geospatial capabilities.
The above is the detailed content of How Can I Insert and Retrieve PostGIS Geometries with Gorm in Golang?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










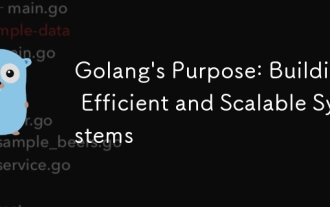
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
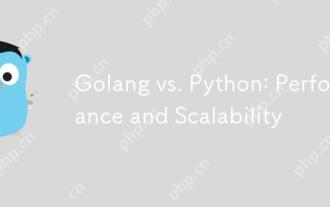
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
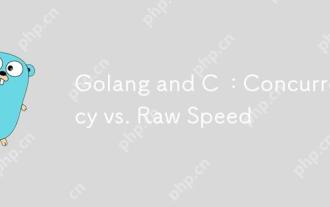
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
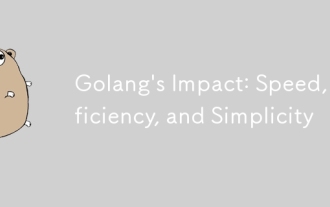
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
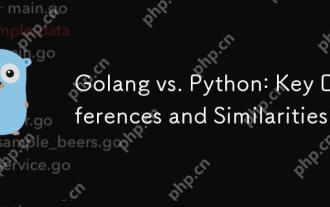
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
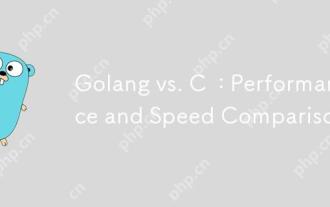
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
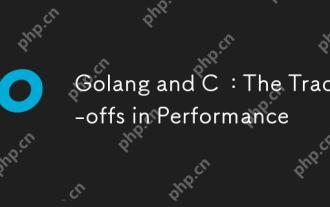
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
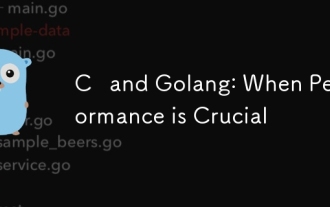
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
