


How do I retrieve all child, grandchild, and descendant nodes under a parent node using PHP with MySQL query results?
Get all Child, Grandchild, etc. Nodes Under Parent Using PHP with MySQL Query Results
Original Issue:
Retrieving all child, grandchild, and subsequent descendant nodes associated with a parent node is a common task when working with hierarchical data structures. This problem arises in scenarios where database tables employ an adjacency list model for data organization.
Approach Using Recursion:
To address this issue, recursion proves to be an effective approach. Here's a detailed explanation of how recursion can be employed to achieve this goal:
1. Establishing a Base Function:
A recursive function is one that calls upon itself to solve a problem and is often used in scenarios involving hierarchical or nested data structures. In this instance, our base function will be named fetch_recursive.
2. Identifying the Criteria for Recursive Calls:
Within fetch_recursive, two primary conditions determine when recursive calls are made:
- Parent Node Found: When the current node being evaluated is the parent node we are interested in (based on the provided ID).
- Child Node Found: When the current node has a parent ID that matches the parent node ID.
3. Constructing the Result Array:
Each time a recursive call is made, the function will populate a result array with relevant data from the current node. This array will grow iteratively as the recursive calls traverse the tree structure.
4. Recursively Searching for Child Nodes:
If the current node has any child nodes (identified by the existence of a children property), another recursive call will be made to retrieve those child nodes. This process continues until all child nodes of the parent node are captured.
Additional Functionality:
1. Handling Grandchildren and Descendants:
The recursive nature of fetch_recursive ensures that it will automatically traverse the hierarchy and retrieve not only child nodes but also grandchildren and subsequent descendants.
2. Unifying Results:
After all recursive calls are complete, the function returns a single, comprehensive array containing all the descendant nodes under the specified parent node.
Code Implementation:
function fetch_recursive($src_arr, $currentid, $parentfound = false, $cats = array()) { foreach($src_arr as $row) { if((!$parentfound && $row['id'] == $currentid) || $row['parent_id'] == $currentid) { $rowdata = array(); foreach($row as $k => $v) $rowdata[$k] = $v; $cats[] = $rowdata; if($row['parent_id'] == $currentid) $cats = array_merge($cats, fetch_recursive($src_arr, $row['id'], true)); } } return $cats; }
Usage:
To utilize the fetch_recursive function, simply pass the original data array (in this case, $data) and the ID of the node from which you want to retrieve the descendants. For instance, to retrieve all child, grandchild, and descendant nodes under node 3:
function fetch_recursive($src_arr, $currentid, $parentfound = false, $cats = array()) { foreach($src_arr as $row) { if((!$parentfound && $row['id'] == $currentid) || $row['parent_id'] == $currentid) { $rowdata = array(); foreach($row as $k => $v) $rowdata[$k] = $v; $cats[] = $rowdata; if($row['parent_id'] == $currentid) $cats = array_merge($cats, fetch_recursive($src_arr, $row['id'], true)); } } return $cats; }
This will populate the $list variable with an array containing all relevant nodes.
The above is the detailed content of How do I retrieve all child, grandchild, and descendant nodes under a parent node using PHP with MySQL query results?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










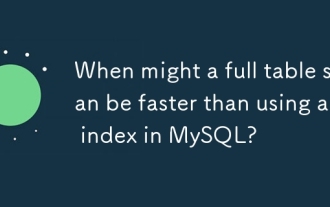
Full table scanning may be faster in MySQL than using indexes. Specific cases include: 1) the data volume is small; 2) when the query returns a large amount of data; 3) when the index column is not highly selective; 4) when the complex query. By analyzing query plans, optimizing indexes, avoiding over-index and regularly maintaining tables, you can make the best choices in practical applications.
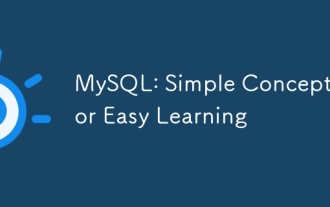
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
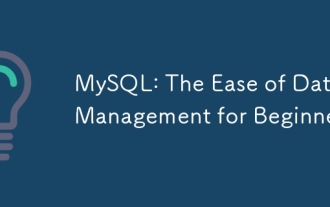
MySQL is suitable for beginners because it is simple to install, powerful and easy to manage data. 1. Simple installation and configuration, suitable for a variety of operating systems. 2. Support basic operations such as creating databases and tables, inserting, querying, updating and deleting data. 3. Provide advanced functions such as JOIN operations and subqueries. 4. Performance can be improved through indexing, query optimization and table partitioning. 5. Support backup, recovery and security measures to ensure data security and consistency.
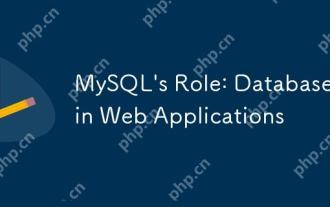
The main role of MySQL in web applications is to store and manage data. 1.MySQL efficiently processes user information, product catalogs, transaction records and other data. 2. Through SQL query, developers can extract information from the database to generate dynamic content. 3.MySQL works based on the client-server model to ensure acceptable query speed.
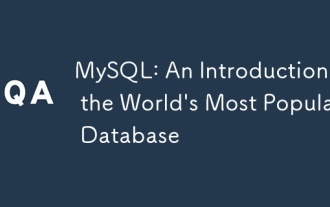
MySQL is an open source relational database management system, mainly used to store and retrieve data quickly and reliably. Its working principle includes client requests, query resolution, execution of queries and return results. Examples of usage include creating tables, inserting and querying data, and advanced features such as JOIN operations. Common errors involve SQL syntax, data types, and permissions, and optimization suggestions include the use of indexes, optimized queries, and partitioning of tables.
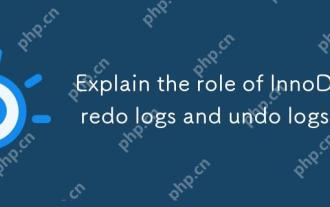
InnoDB uses redologs and undologs to ensure data consistency and reliability. 1.redologs record data page modification to ensure crash recovery and transaction persistence. 2.undologs records the original data value and supports transaction rollback and MVCC.
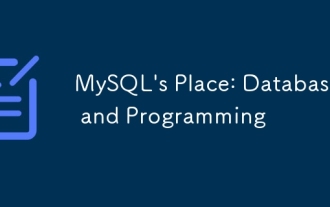
MySQL's position in databases and programming is very important. It is an open source relational database management system that is widely used in various application scenarios. 1) MySQL provides efficient data storage, organization and retrieval functions, supporting Web, mobile and enterprise-level systems. 2) It uses a client-server architecture, supports multiple storage engines and index optimization. 3) Basic usages include creating tables and inserting data, and advanced usages involve multi-table JOINs and complex queries. 4) Frequently asked questions such as SQL syntax errors and performance issues can be debugged through the EXPLAIN command and slow query log. 5) Performance optimization methods include rational use of indexes, optimized query and use of caches. Best practices include using transactions and PreparedStatemen
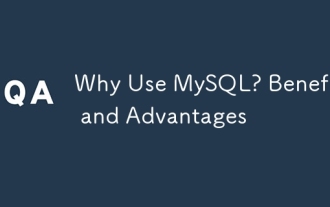
MySQL is chosen for its performance, reliability, ease of use, and community support. 1.MySQL provides efficient data storage and retrieval functions, supporting multiple data types and advanced query operations. 2. Adopt client-server architecture and multiple storage engines to support transaction and query optimization. 3. Easy to use, supports a variety of operating systems and programming languages. 4. Have strong community support and provide rich resources and solutions.
