


How to Switch Between Multiple Browser Windows Using Selenium in Python?
How to Manage Multiple Browser Windows Using Selenium in Python
In the vast realm of web automation, it is often necessary to interact with multiple browser windows or tabs. Selenium, an industry-leading automation framework, empowers Python developers with the ability to navigate these challenges seamlessly.
One common scenario encountered during web testing is the opening of a new browser window upon clicking a link. To effectively perform actions within the newly opened window, we must switch the focus away from the background window.
Finding the Target Window's Handle
Before switching to the new window, we need to identify its handle. This unique identifier represents the specific window instance. To retrieve the handles, we utilize the driver.window_handles method, which returns a list of all open window handles. The handle of the currently focused window will be the first element in this list.
Switching to the New Window
Now that we have the handle of the target window, we can switch to it using the driver.switch_to.window(handle) method. Passing in the target handle effectively moves the focus to the corresponding window, allowing us to perform actions within its context.
Code Example
The following Python code illustrates how to switch to a newly opened window:
import unittest from selenium import webdriver class GoogleOrgSearch(unittest.TestCase): def setUp(self): self.driver = webdriver.Firefox() def test_google_search_page(self): driver = self.driver driver.get("http://www.cdot.in") window_before = driver.window_handles[0] print(window_before) driver.find_element_by_xpath("//a[@href='http://www.cdot.in/home.htm']").click() window_after = driver.window_handles[1] driver.switch_to.window(window_after) print(window_after) driver.find_element_by_link_text("ATM").click() driver.switch_to.window(window_before) def tearDown(self): self.driver.close() if __name__ == "__main__": unittest.main()
In this example, we are accessing the "http://www.cdot.in" website and clicking a link to open a new window. We then retrieve the handles of the two windows and switch to the newly opened one to perform further actions.
The above is the detailed content of How to Switch Between Multiple Browser Windows Using Selenium in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










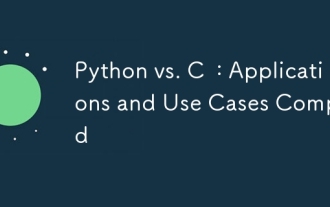
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
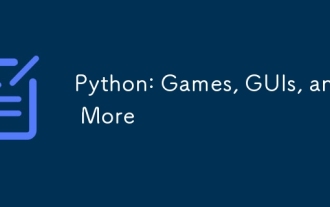
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
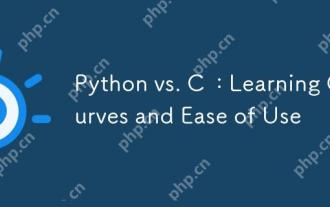
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
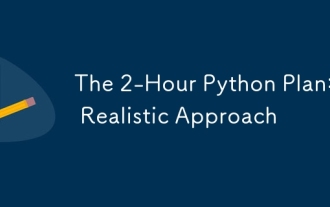
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
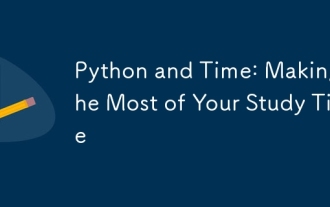
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
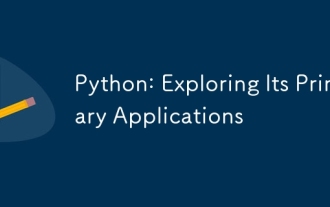
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
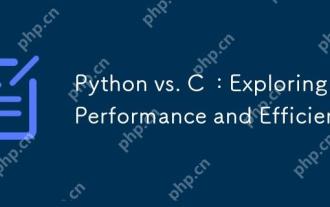
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.
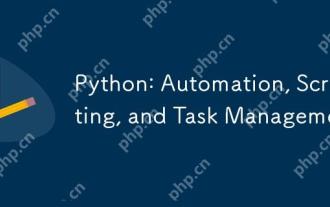
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
