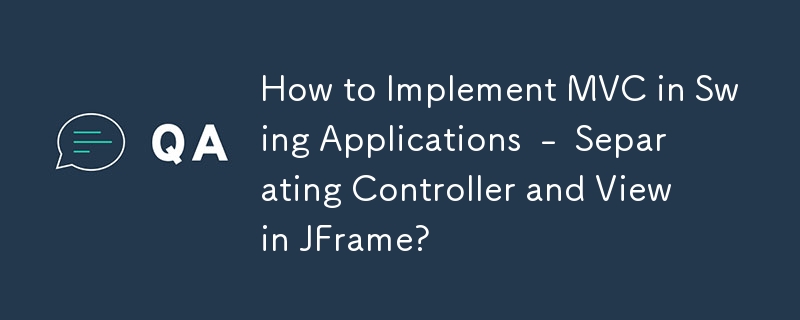
MVC in Swing: Clarifying the Confusion
Dilemma of Mixing Controller and View in Swing Applications
In Swing applications, implementing the Model-View-Controller (MVC) pattern can be challenging, particularly when it comes to associating controller functionality within the JFrame. This issue arises when actions that handle field clearing, validation, locking, and button interactions are directly placed within the JFrame, potentially mixing the Controller and View responsibilities.
Understanding the MVC Paradigm
To clarify this confusion, it is helpful to revisit the core principles of the MVC pattern:
-
View: The interface that handles user interaction and displays data from the Model.
-
Model: The underlying data that is independent of the View.
-
Controller: The intermediary between the View and Model, responsible for processing user input and updating the Model accordingly, while potentially influencing the View's presentation.
Applying MVC in a JFrame Context
In the context of a Swing JFrame, consider the following guidelines:
- Create a separate class to represent the View, containing the JTable, text fields, and buttons.
- Use a TableModel to bridge the JTable with the Model, facilitating data retrieval and display.
- Create a Controller class that interacts with the Model and View.
- Within the View (JFrame), register an ActionListener to capture user interactions.
- When an event is triggered, the View invokes the appropriate method in the Controller.
- The Controller processes the event, updates the Model as needed, and may request the View to change its presentation (e.g., disabling buttons).
- The Model notifies its observers (including the View) when it changes, prompting the View to update its display.
Additional Considerations
- One crucial consideration is managing the interplay between SwingWorker and EventDispatch threads within the MVC pattern.
- Consider using composite patterns such as the Observer and Strategy patterns in your implementation for improved modularity.
By adhering to these principles, you can effectively separate Controller and View responsibilities, maintaining the integrity of the MVC design pattern in your Swing applications.
The above is the detailed content of How to Implement MVC in Swing Applications - Separating Controller and View in JFrame?. For more information, please follow other related articles on the PHP Chinese website!