


Why is it difficult to log in to Barnes & Noble's mobile site using Curl, SSL, and cookies?
How to Log In with Curl, SSL, and Cookies
When attempting to log into barnesandnoble.com using Curl, SSL, and cookies, some users encounter difficulties due to differences in website protocols and potential cookie handling issues. Here's a breakdown of the problem and a solution:
Problem:
- Users are unable to log into barnesandnoble.com's mobile site using Curl.
- The returned page displays no errors, and the email field defaults to the login form's email input box.
- The same code successfully logs into eBay, indicating that the issue lies in the barnesandnobles website's unique characteristics.
Solution:
To successfully log into barnesandnoble.com using Curl, consider the following:
- URL Encoding: Ensure that URL parameters (such as email and password) in the post string are properly URL-encoded.
- x Value: Include the x value as part of the login URL. This value is typically extracted from the initial response.
Here's an example script that incorporates these solutions:
// Options $EMAIL = '[email protected]'; $PASSWORD = 'yourpassword'; $cookie_file_path = "/tmp/cookies.txt"; $LOGINURL = "https://cart2.barnesandnoble.com/mobileacct/op.asp?stage=signIn"; $agent = "Nokia-Communicator-WWW-Browser/2.0 (Geos 3.0 Nokia-9000i)"; // Begin Script $ch = curl_init(); // Extra Headers $headers[] = "Accept: */*"; $headers[] = "Connection: Keep-Alive"; // Basic Curl Options curl_setopt($ch, CURLOPT_HTTPHEADER, $headers); curl_setopt($ch, CURLOPT_HEADER, 0); curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, 0); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false); curl_setopt($ch, CURLOPT_USERAGENT, $agent); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); curl_setopt($ch, CURLOPT_FOLLOWLOCATION, 1); curl_setopt($ch, CURLOPT_COOKIEFILE, $cookie_file_path); curl_setopt($ch, CURLOPT_COOKIEJAR, $cookie_file_path); // Initial URL curl_setopt($ch, CURLOPT_URL, $LOGINURL); // Get Cookies and Form Inputs $content = curl_exec($ch); // Extract Hidden Inputs $fields = getFormFields($content); $fields['emailAddress'] = $EMAIL; $fields['acctPassword'] = $PASSWORD; // Get x Value $x = ''; if (preg_match('/op\.asp\?x=(\d+)/i', $content, $match)) { $x = $match[1]; } // Updated Login URL $LOGINURL = "https://cart2.barnesandnoble.com/mobileacct/op.asp?x=$x"; // Post Options curl_setopt($ch, CURLOPT_URL, $LOGINURL); curl_setopt($ch, CURLOPT_POST, 1); curl_setopt($ch, CURLOPT_POSTFIELDS, http_build_query($fields)); // Perform Login $result = curl_exec($ch); print $result; // Helper Function to Extract Form Inputs function getFormFields($data) { $inputs = array(); $elements = preg_match_all('/(<input[^>]+>)/is', $data, $matches); if ($elements > 0) { for ($i = 0; $i < $elements; $i++) { $el = preg_replace('/\s{2,}/', ' ', $matches[1][$i]); if (preg_match('/name=(?:["\'])?([^"\'\s]*)/i', $el, $name)) { $name = $name[1]; $value = ''; if (preg_match('/value=(?:["\'])?([^"\'\s]*)/i', $el, $value)) { $value = $value[1]; } $inputs[$name] = $value; } } } return $inputs; }
Remember to modify the $EMAIL and $PASSWORD variables with your actual login credentials. Additionally, the $cookie_file_path should point to a writable location on the file system.
Once you have logged in, you can create a new Curl object, specifying the COOKIEFILE and COOKIEJAR options, and you will remain authenticated without performing the initial steps.
The above is the detailed content of Why is it difficult to log in to Barnes & Noble's mobile site using Curl, SSL, and cookies?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










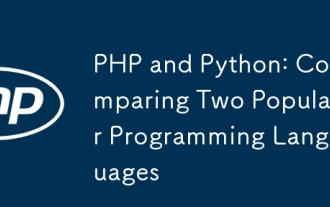
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
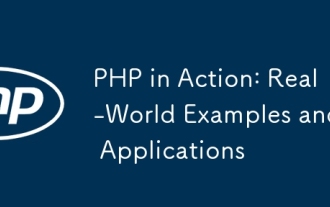
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
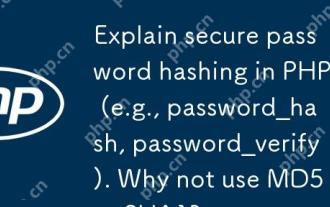
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
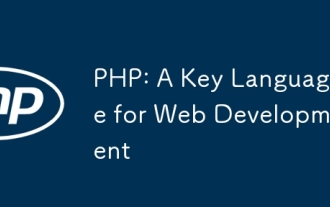
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
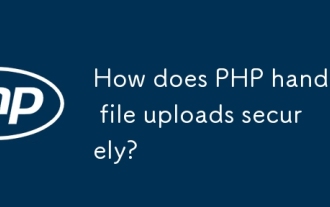
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
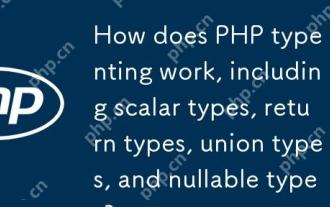
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
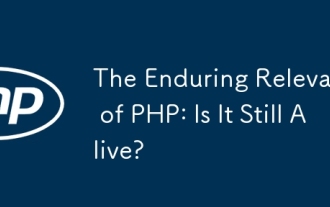
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
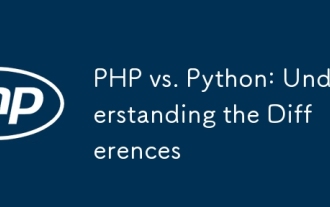
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
