


How Can I Efficiently Send and Receive 2D Arrays Between Nodes Using MPI?
Sending and Receiving 2D Array over MPI
Utilizing MPI for parallel processing offers immense performance advantages, especially for computations involving large matrices. In such scenarios, splitting a matrix across multiple nodes can significantly optimize the process.
Implementing Edge Value Sharing
In the provided scenario, each node must share edge values with its neighbors. The suggested scheme to achieve this using MPI is as follows:
if (myrank == 0) { for (i = 0 to x) { for (y = 0 to y) { C++ CODE IMPLEMENTATION MPI_SEND(A[x][0], A[x][1], A[x][2], Destination= 1...) MPI_RECEIVE(B[0][0], B[0][1]......Sender = 1...) } } if (myrank == 1) { for (i = x+1 to xx) { for (y = 0 to y) { C++ CODE IMPLEMENTATION MPI_SEND(B[x][0], B[x][1], B[x][2], Destination= 0...) MPI_RECEIVE(A[0][0], A[0][1]......Sender = 1...) } } }
Optimizing Array Allocation
To simplify memory management and MPI communication, consider allocating arrays with contiguous elements instead of C's "multidimensional arrays." This can be achieved using functions like:
int **alloc_2d_int(int rows, int cols) { int *data = (int *)malloc(rows*cols*sizeof(int)); int **array= (int **)malloc(rows*sizeof(int*)); for (int i=0; i<rows; i++) array[i] = &(data[cols*i]); return array; } int **A; A = alloc_2d_init(N,M);
MPI Send/Receive
Once the arrays are allocated contiguously, sending and receiving entire N x M arrays becomes straightforward:
MPI_Send(&(A[0][0]), N*M, MPI_INT, destination, tag, MPI_COMM_WORLD);
Barriers vs. Blocking Sends/Receives
MPI offers multiple functions for communication, including blocking (e.g., MPI_Send) and non-blocking (e.g., MPI_Isend). For blocking operations, barriers are unnecessary, as communication is inherently synchronized.
Other MPI Functions
In addition to MPI_Send and MPI_Receive, consider using MPI_Sendrecv for more flexible communication or non-blocking operations like MPI_Isend and MPI_Irecv to overlap communication and computation.
The above is the detailed content of How Can I Efficiently Send and Receive 2D Arrays Between Nodes Using MPI?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
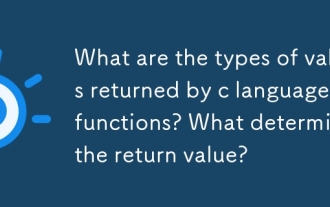
What are the types of values returned by c language functions? What determines the return value?
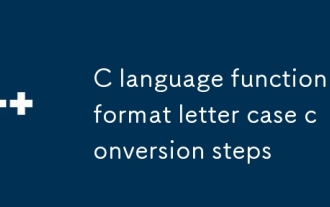
C language function format letter case conversion steps

What are the definitions and calling rules of c language functions and what are the

Where is the return value of the c language function stored in memory?
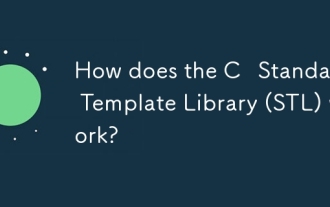
How does the C Standard Template Library (STL) work?
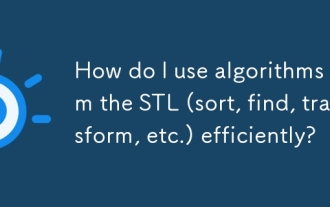
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
