How to Index Multiple Arrays in NumPy?
Indexing Multiple Arrays in NumPy
In NumPy, indexing beyond single-dimensional arrays requires advanced techniques. One scenario is indexing one array based on the values of another array, known as multidimensional indexing.
Consider matrices A with arbitrary values:
array([[ 2, 4, 5, 3], [ 1, 6, 8, 9], [ 8, 7, 0, 2]])
And matrix B containing indices of elements in A:
array([[0, 0, 1, 2], [0, 3, 2, 1], [3, 2, 1, 0]])
To select values from A using the indices in B, you can employ NumPy's advanced indexing:
A[np.arange(A.shape[0])[:,None],B]
This indexing approach combines the row indices (0, 1, 2) with the indices specified in B.
Alternatively, you can use linear indexing:
m,n = A.shape out = np.take(A,B + n*np.arange(m)[:,None])
Here, m and n represent the number of rows and columns in A, respectively. np.take() extracts elements from A based on the linear indices generated by the sum of B and n multiplied by the range of row indices.
Using either technique, the output will be:
[[2, 2, 4, 5], [1, 9, 8, 6], [2, 0, 7, 8]]
This indexing method provides flexibility in accessing and manipulating elements based on multiple criteria, enhancing the versatility of NumPy arrays for complex data processing scenarios.
The above is the detailed content of How to Index Multiple Arrays in NumPy?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










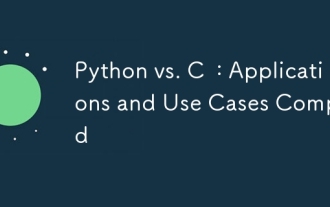
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
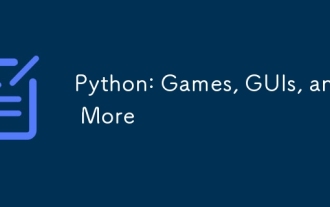
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
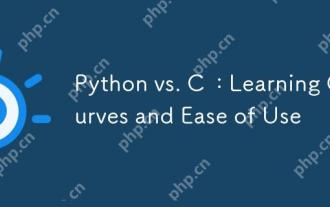
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
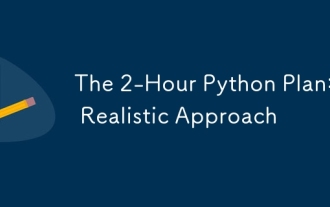
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
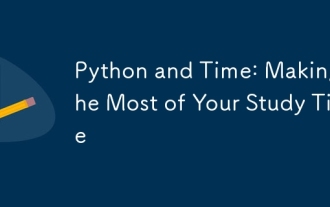
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
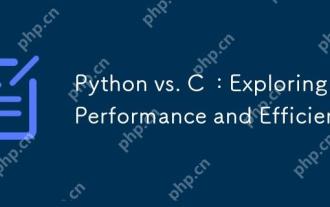
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.
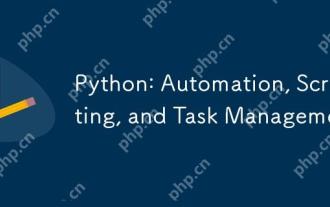
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
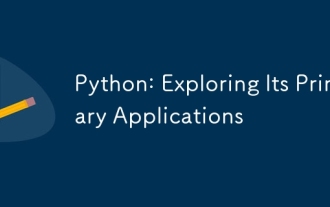
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
