


When Should You Use 'try' vs. 'if' to Test Variable Values in Python?
Nov 10, 2024 am 01:28 AMUsing "try" vs. "if" to Test Variable Value in Python
In Python, there are situations where you may need to check if a variable has a value before processing it. This dilemma arises when deciding between using "if" or "try" constructs.
"if" Constructs
The "if" statement checks if a condition is True and executes the indented block of code if it is. In your example, using an "if" construct would look like this:
result = function() if result: for r in result: # Process items
This approach assumes that the result variable holds a non-empty value. If result is an empty list or None, an IndexError or TypeError will be raised when trying to iterate over result.
"try" Constructs
A "try" block attempts to execute a block of code and catches any exceptions that may occur. In this case, you can use a try/except block to handle exceptions gracefully:
result = function() try: for r in result: # Process items except TypeError: pass
This code will attempt to iterate over the result variable. If it encounters a TypeError due to an empty list or None, it will skip the error and continue execution.
Choosing Between "if" and "try"
The choice between using "if" and "try" depends on the specific situation and the likelihood of an exception being raised.
-
Use "if" constructs when:
- You have a strong expectation that the variable will have a non-empty value.
- Performance is critical, as "if" checks are faster than "try" blocks.
-
Use "try" constructs when:
- You expect the variable to be empty or null in some cases.
- You want to handle exceptions gracefully without interrupting the program flow.
- EAFP Style
Python encourages the "EAFP" (Easier to Ask for Forgiveness Than Permission) style, where exceptions are handled after attempting operations that may fail. This approach can be more efficient and concise, as seen in the "try" example above.
The above is the detailed content of When Should You Use 'try' vs. 'if' to Test Variable Values in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
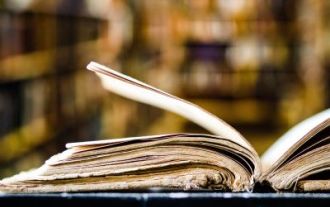
How to Use Python to Find the Zipf Distribution of a Text File
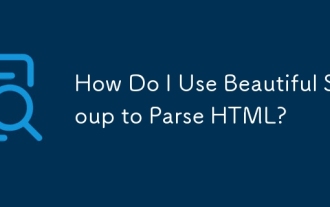
How Do I Use Beautiful Soup to Parse HTML?
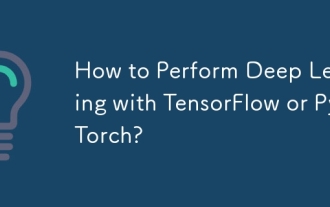
How to Perform Deep Learning with TensorFlow or PyTorch?
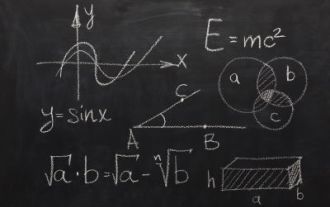
Mathematical Modules in Python: Statistics
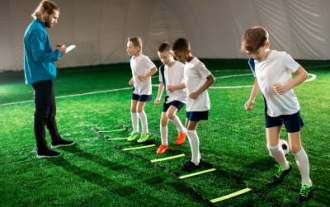
Introduction to Parallel and Concurrent Programming in Python
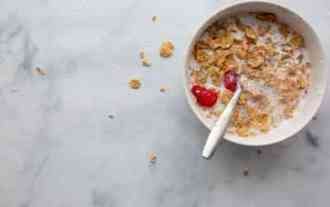
Serialization and Deserialization of Python Objects: Part 1
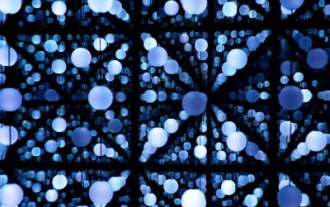
How to Implement Your Own Data Structure in Python
