


Can You Pass Multiple Variables in a Request Body to a Spring MVC Controller Using Ajax?
Passing Multiple Variables in @RequestBody to a Spring MVC Controller Using Ajax
When attempting to pass multiple variables in a request body to a Spring MVC controller using Ajax, you may face the question of whether it is necessary to enclose the variables within a backing object.
The original query expressed a desire to achieve this using the following approach:
@RequestMapping(value = "/Test", method = RequestMethod.POST) @ResponseBody public boolean getTest(@RequestBody String str1, @RequestBody String str2) {}
With JSON data resembling:
{ "str1": "test one", "str2": "two test" }
However, the author faced the need to use a holder object instead:
@RequestMapping(value = "/Test", method = RequestMethod.POST) @ResponseBody public boolean getTest(@RequestBody Holder holder) {}
And accompanying JSON:
{ "holder": { "str1": "test one", "str2": "two test" } }
The dilemma lies in the fact that @RequestBody requires mapping to a single object, making the use of multiple parameters in the request body challenging.
While the aforementioned workaround involving a holder object is a valid solution, an alternative approach is available using a Map object:
@RequestMapping(value = "/Test", method = RequestMethod.POST) @ResponseBody public boolean getTest(@RequestBody Map<String, String> json) { //json.get("str1") == "test one" }
This method provides a flexible way to handle multiple variables passed in the request body, allowing access to them via map keys.
Additionally, if you prefer to bind to Jackson's ObjectNode to work with a full JSON tree, you can utilize the following:
public boolean getTest(@RequestBody ObjectNode json) { //json.get("str1").asText() == "test one" }
These alternatives empower you with multiple options for handling multiple variables in a request body, giving you the flexibility to choose the approach that best suits your specific needs.
The above is the detailed content of Can You Pass Multiple Variables in a Request Body to a Spring MVC Controller Using Ajax?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










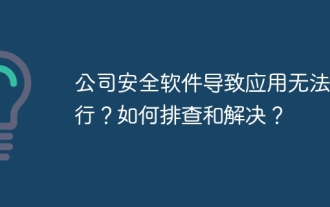
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
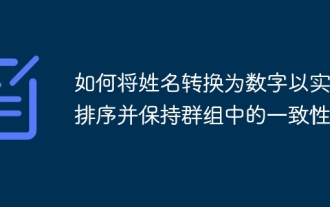
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
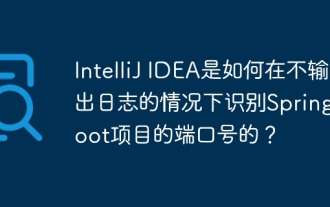
Start Spring using IntelliJIDEAUltimate version...
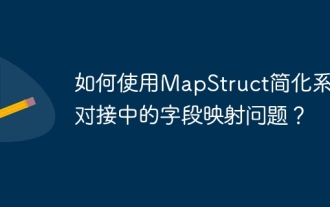
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
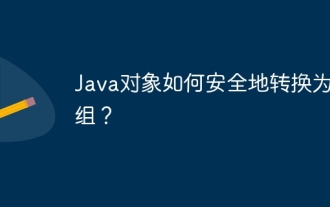
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
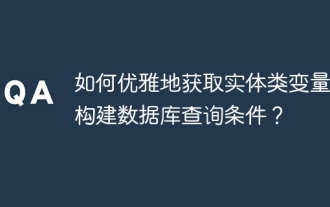
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
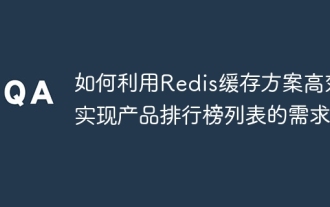
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
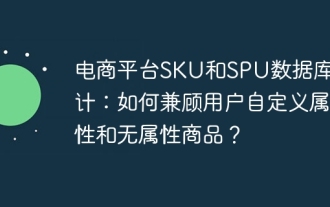
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
