How to Dereference Pointer Fields for Effective Debugging in Go?
Dereferencing Pointer Fields for Debugging
When printing a struct with pointer fields, they are usually displayed as memory addresses. This can be inconvenient for debugging, especially if a struct contains numerous pointer fields.
Consider this example:
package main import "fmt" type SomeStruct struct { somePointer *somePointer } type somePointer struct { field string } func main() { fmt.Println(SomeStruct{&somePointer{"I want to see what is in here"}}) }
This code prints the memory address instead of the desired value:
{0x10500168}
To print the actual value stored in the pointer field, we can use the go-spew package, which specializes in printing complex data structures in a human-readable format.
Here's how to use it:
package main import ( "github.com/davecgh/go-spew/spew" ) type ( SomeStruct struct { Field1 string Field2 int Field3 *somePointer } somePointer struct { field string } ) func main() { s := SomeStruct{ Field1: "Yahoo", Field2: 500, Field3: &somePointer{"I want to see what is in here"}, } spew.Dump(s) }
This code produces the following output:
(main.SomeStruct) { Field1: (string) "Yahoo", Field2: (int) 500, Field3: (*main.somePointer)(0x2102a7230)({ field: (string) "I want to see what is in here" }) }
As you can see, go-spew provides a much more detailed and informative representation of the struct. The pointer fields are dereferenced and displayed as their actual values. This makes it much easier to inspect the content of a struct during debugging.
The above is the detailed content of How to Dereference Pointer Fields for Effective Debugging in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










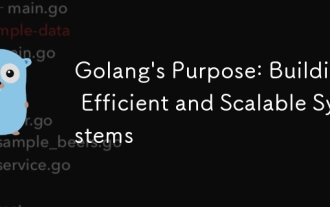
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
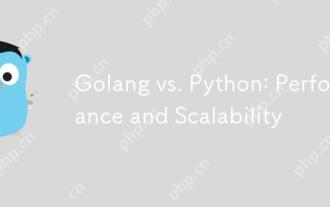
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
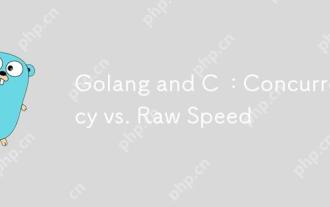
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
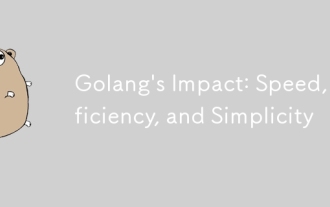
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
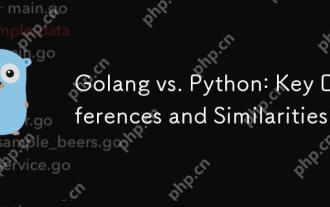
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
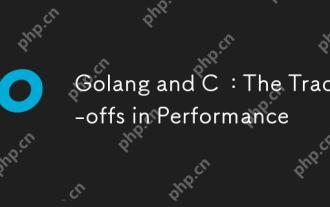
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
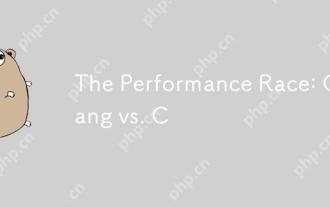
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
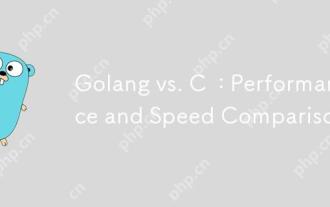
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
