


Why is my FastAPI StreamingResponse not streaming with a generator function?
FastAPI StreamingResponse Not Streaming with Generator Function
Problem:
A FastAPI application fails to stream a response from a generator function using StreamingResponse, resulting in the entire response being sent as a whole.
Answer:
There are several factors to consider when using StreamingResponse with generator functions:
1. HTTP Request Type:
The provided code uses a POST request, which is not suitable for obtaining data from the server. Use a GET request instead to fetch data.
2. Credential Handling:
For security reasons, avoid sending credentials (e.g., 'auth_key') via the URL query string. Use headers or cookies instead.
3. Generator Function Syntax:
Blocking operations should not be executed within the generator function of StreamingResponse. Use def instead of async def for the generator function, as FastAPI uses a thread pool to manage blocking operations.
4. Iterator Usage:
In your test code, requests.iter_lines() iterates through the response data one line at a time. If the response does not contain line breaks, use iter_content() and specify a chunk size to avoid potential buffering issues.
5. Media Type:
Browsers may buffer responses with media_type='text/plain'. To avoid this, set media_type='text/event-stream' or disable MIME Sniffing using X-Content-Type-Options: nosniff in the response headers.
Working Example:
Here is a working example in app.py and test.py that addresses the issues mentioned above:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
|
The above is the detailed content of Why is my FastAPI StreamingResponse not streaming with a generator function?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










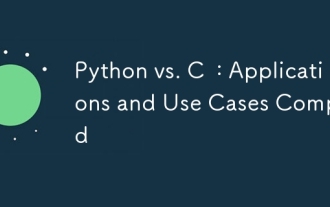
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
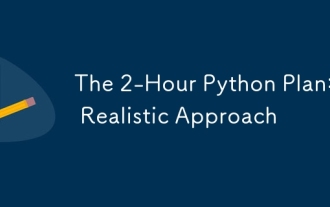
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
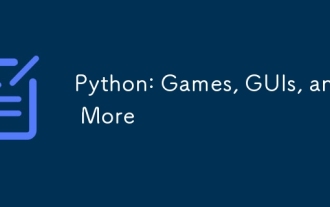
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
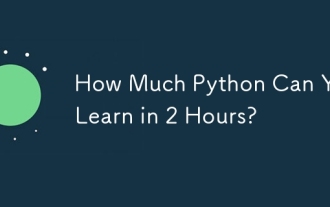
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
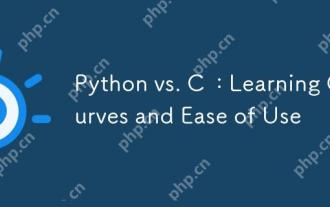
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
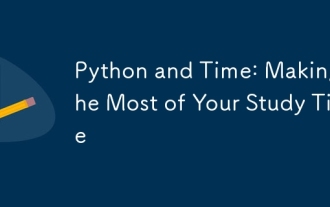
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
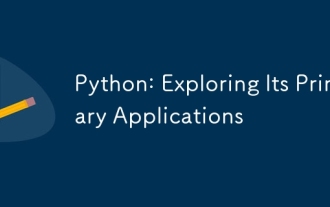
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
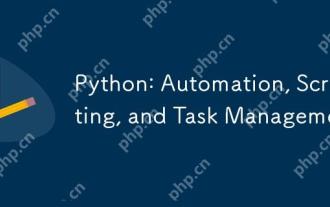
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
