Random Access Files
Java allows direct access to any position in a file with the RandomAccessFile class, ideal for manipulating data that needs to be read or written out of order. Unlike the InputStream and OutputStream classes, RandomAccessFile implements the DataInput and DataOutput interfaces, which provide methods for manipulating primitive data types (such as int, double, etc.).
RandomAccessFile Structure and Builder
Constructor:
RandomAccessFile(String nomeArquivo, String acesso)
- filename: file name.
- access: defines the type of access ("r" for reading, "rw" for reading and writing).
- Seek(long position) method: Defines the position of the pointer within the file.
void seek(long novaPos) throws IOException
- novaPos: Position in bytes from the beginning of the file. After seek, reading/writing happens from the new position.
Code Example: Random Access to Double Values
Below, an example that writes six double values to a file and then reads them at specific positions.
import java.io.*; class RandomAccessDemo { public static void main(String args[]) { double data[] = { 19.4, 10.1, 123.54, 33.0, 87.9, 74.25 }; double d; // Abre e usa um arquivo de acesso aleatório try (RandomAccessFile raf = new RandomAccessFile("random.dat", "rw")) { // Grava os valores no arquivo for(int i = 0; i < data.length; i++) { raf.writeDouble(data[i]); } // Lê valores específicos com o método seek() raf.seek(0); // Busca o primeiro valor d = raf.readDouble(); System.out.println("Primeiro valor: " + d); raf.seek(8); // Busca o segundo valor d = raf.readDouble(); System.out.println("Segundo valor: " + d); raf.seek(8 * 3); // Busca o quarto valor d = raf.readDouble(); System.out.println("Quarto valor: " + d); System.out.println("\nValores alternados:"); for(int i = 0; i < data.length; i += 2) { raf.seek(8 * i); // Busca o i-ésimo valor d = raf.readDouble(); System.out.print(d + " "); } } catch(IOException exc) { System.out.println("Erro de I/O: " + exc); } } }
Code Explanation:
Writing: Double values are written to the random.dat file. As each double occupies 8 bytes, each value starts in multiples of 8 bytes (0, 8, 16, ...).
Reading with seek():
- First value: Starts at byte 0.
- Second value: Starts at byte 8.
- Fourth value: Starts at byte 24 (3rd index, since 8 * 3 = 24).
- Alternating values: Every 16 bytes (8 bytes between each value), the values are read.
Code Output
Example output:
Primeiro valor: 19.4 Segundo valor: 10.1 Quarto valor: 33.0 Valores alternados: 19.4 123.54 87.9
Important Points:
Positioning with seek(): Defines the position of the pointer to read/write anywhere in the file.
Position Control with Bytes: As each double occupies 8 bytes, we can calculate the position of each value using multiples of 8.
This example is useful for handling large amounts of data efficiently, accessing only the necessary points without traversing the entire file.
The above is the detailed content of Random Access Files. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










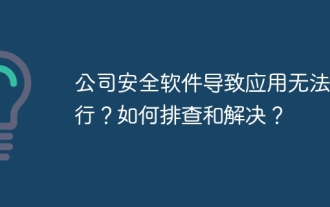
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
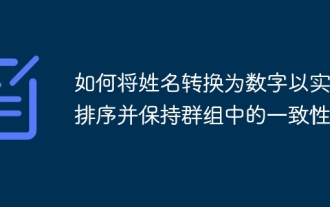
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
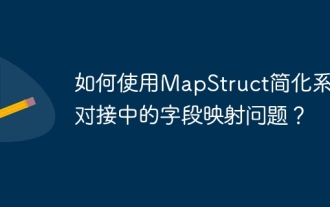
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
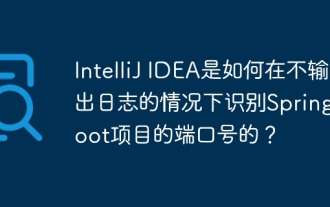
Start Spring using IntelliJIDEAUltimate version...
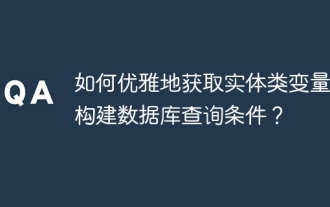
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
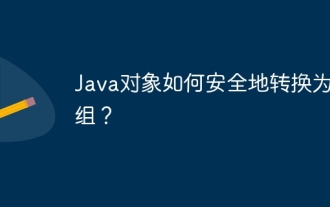
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
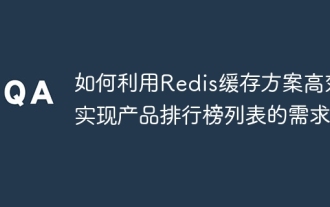
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
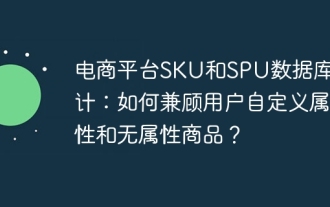
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
