


Why Do Callback Functions Sometimes Execute Asynchronously, Despite No Explicit Syntax?
Why Do Callback Functions Execute Asynchronously?
In the world of programming, callback functions are a crucial part of asynchronous programming. But what exactly in the syntax triggers their asynchronous execution? Surprisingly, the answer lies not in the syntax itself, but in the underlying implementation details.
Decoding the Non-Blocking Nature of Callbacks
برخلاف تصور عمومی، nothing in the syntax of a callback function explicitly declares it as asynchronous. Take the following examples:
setTimeout(function() { console.log("Asynchronous Callback"); }, 100);
my_array.forEach(function(element) { console.log("Synchronous Callback"); });
Both examples use callback functions, but only the first one is asynchronous, delayed by 100 milliseconds using setTimeout. The second one executes synchronously, immediately iterating through the array.
The only reliable method to determine a callback's behavior is to consult the documentation or perform a test to verify its execution time.
The Magic Behind Asynchronous Functions
Javascript, as a language, doesn't inherently provide asynchronous execution for functions. To achieve this, either another asynchronous function (like setTimeout or web workers) is utilized, or the function is written in C.
C-coded functions, such as setTimeout, implement asynchronicity through the event loop.
The Event Loop and Asynchronous Execution
The event loop is a fundamental part of a web browser's architecture. It handles I/O operations in a non-blocking manner, allowing multiple tasks to occur concurrently.
The event loop primarily relies on select() or similar functions in C to monitor I/O events. When data becomes available, the interpreter calls the appropriate callback associated with that I/O channel.
Timeout Management and Web Workers
The event loop seamlessly handles timeout events and web workers. By managing timeouts passed to select(), it can schedule callbacks to execute in the future. Web workers, which run on separate threads, also interact with the event loop to communicate with the main thread.
Additional Resources
For a deeper understanding of non-blocking I/O programming in C, refer to: http://www.gnu.org/software/libc/manual/html_node/Waiting-for-I_002fO.html
Explore the following articles for further insights:
- Is nodejs representing Reactor or Proactor design pattern?
- Performance of NodeJS with large amount of callbacks
The above is the detailed content of Why Do Callback Functions Sometimes Execute Asynchronously, Despite No Explicit Syntax?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










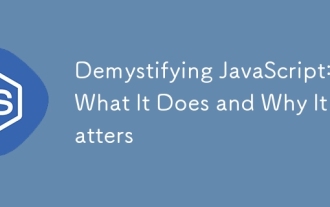
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
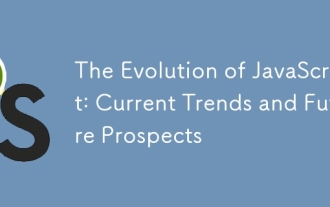
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
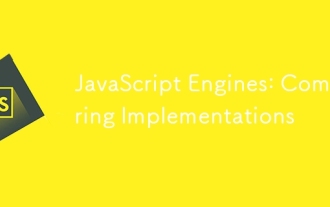
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
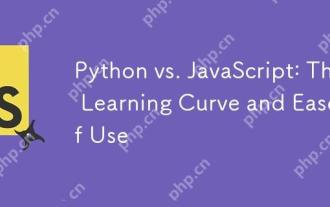
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
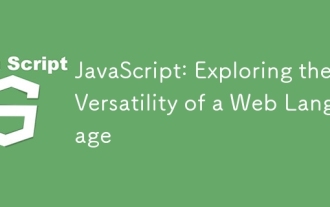
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
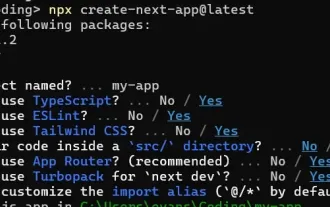
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
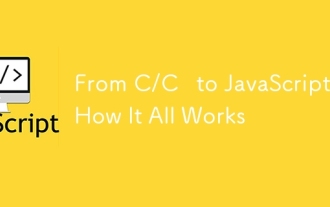
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
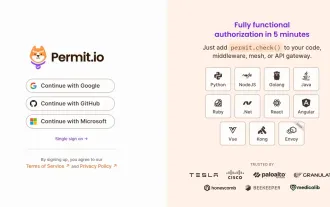
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
