How to Concatenate PEM Certificates for HTTPS in Go Web Servers?
Concatenating PEM Certificates for HTTPS in Go Web Server
When setting up HTTPS for a Go web server, you often encounter a need to concatenate multiple PEM certificate files. This process is essential to create a complete certificate chain that includes both your primary certificate and intermediate certificates.
Obtaining Intermediate Certificates
Typically, you will be provided with a bundle of PEM files when you purchase an SSL certificate, including:
- csr.pem (ignored)
- private-key.pem (private key)
- website.com.crt (primary certificate)
- website.com.ca-bundle (intermediate certificates)
- website.com.zip (zipped version of the bundle)
Concatenating the Certificates
As per the documentation at https://www.kaihag.com/https-and-go/, you need to concatenate the following PEM files:
- website.com.ca-bundle (intermediate certificate(s))
- website.com.crt (primary certificate)
To do this, you can use the following command (assuming the files are in the current directory):
cat website.com.ca-bundle website.com.crt > full-cert.crt
This creates a combined certificate file named full-cert.crt, which contains both the intermediate certificates and your primary certificate.
Setting Up HTTPS in Go
Once you have a concatenated certificate file, you can use the http.ListenAndServeTLS function to start your HTTPS server in Go. Here's an example code snippet:
package main import ( "log" "net/http" ) func handler(w http.ResponseWriter, r *http.Request) { w.Write([]byte("Hello, HTTPS!")) } func main() { log.Printf("About to listen on 10443. Go to https://127.0.0.1:10443/") err := http.ListenAndServeTLS(":10443", "full-cert.crt", "private-key.pem", nil) log.Fatal(err) }
Replace full-cert.crt with the name of your concatenated certificate file and private-key.pem with the name of your private key file. Run the program and access https://127.0.0.1:10443/ to confirm that HTTPS is working correctly.
The above is the detailed content of How to Concatenate PEM Certificates for HTTPS in Go Web Servers?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










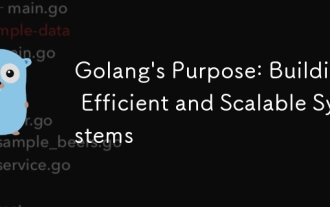
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
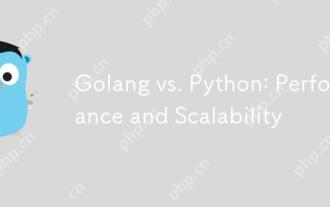
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
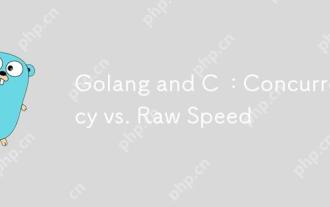
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
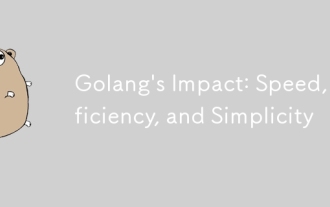
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
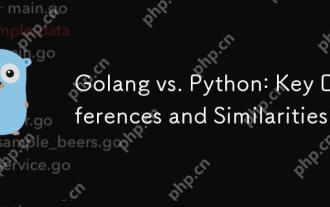
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
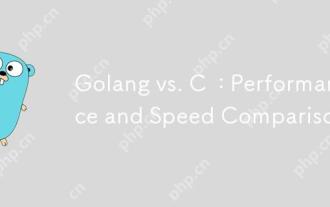
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
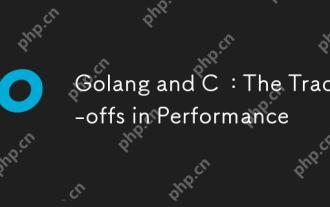
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
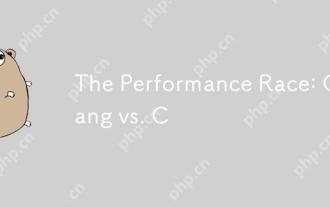
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
