


How can you ensure that all arguments passed to a variadic function or template function are of the same type without using additional data structures?
Specifying One Type for Variadic Arguments
This article explores a method to ensure that all arguments passed to a variadic function or variadic template function are of the same type, without using additional data structures such as arrays, vectors, or structs.
Variadic Functions and Template Functions
Variadic functions and variadic template functions allow a function to accept an unknown number of arguments. However, they do not enforce any type constraints on the arguments.
Enforcing Type Safety
To guarantee that all arguments are of the same type, we can take the following steps:
- Accept Arguments by Variadic Template: Define the function or template function to accept arguments by a variadic template parameter.
- Use SFINAE for Convertibility Check: Use the Substitution Failure Is Not an Error (SFINAE) technique on the function interface to check if the arguments can be converted to a specific type. This technique allows us to reject invalid arguments early.
- Define a Helper Type: Create a helper type using a variadic template fst (First Type) to determine the type of the first argument.
- Apply SFINAE to the Function Signature: Apply enable_if to the function signature to check if the arguments are convertible to the desired type. If the check fails, the compiler will generate an error.
Example:
The following code demonstrates how to implement this technique:
template<typename R, typename...> struct fst { typedef R type; }; template<typename ...Args> typename fst<void, typename enable_if< is_convertible<Args, ToType>::value >::type... >::type f(Args...);
Usage:
This code ensures that all arguments passed to f can be converted to the ToType type. If any argument is not convertible, the compiler will issue an error.
Convert-Later Approach:
Alternatively, if you know the steps to convert from an array to the desired type, you can use the following approach:
template<typename ...Items> dragon_list_t make_dragon_list(Items... maidens) { std::array<Maiden, sizeof...(Items)> arr = {{ maidens ... }}; // here be dragons }
Conclusion:
By utilizing variadic template functions and SFINAE, we can enforce type safety on variadic arguments, ensuring that all arguments are of the same type. This allows us to create functions that operate on homogeneous data without the need for additional data structures.
The above is the detailed content of How can you ensure that all arguments passed to a variadic function or template function are of the same type without using additional data structures?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




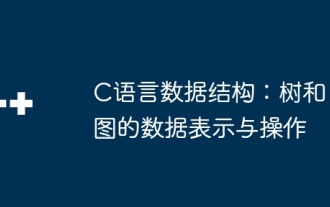
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
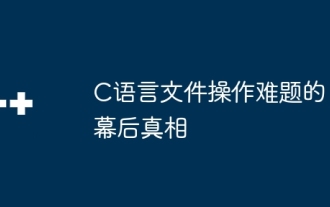
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
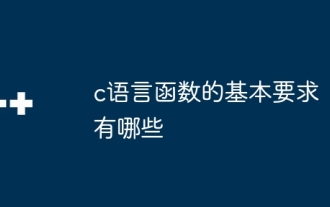
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
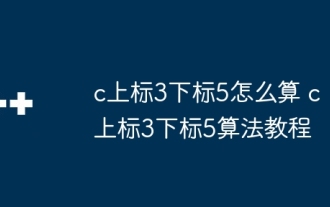
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
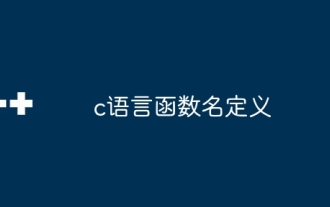
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
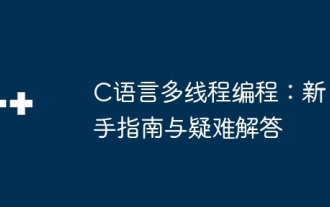
C language multithreading programming guide: Creating threads: Use the pthread_create() function to specify thread ID, properties, and thread functions. Thread synchronization: Prevent data competition through mutexes, semaphores, and conditional variables. Practical case: Use multi-threading to calculate the Fibonacci number, assign tasks to multiple threads and synchronize the results. Troubleshooting: Solve problems such as program crashes, thread stop responses, and performance bottlenecks.
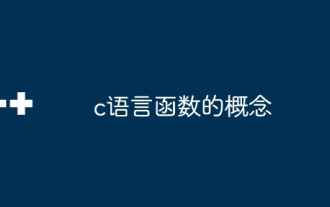
C language functions are reusable code blocks. They receive input, perform operations, and return results, which modularly improves reusability and reduces complexity. The internal mechanism of the function includes parameter passing, function execution, and return values. The entire process involves optimization such as function inline. A good function is written following the principle of single responsibility, small number of parameters, naming specifications, and error handling. Pointers combined with functions can achieve more powerful functions, such as modifying external variable values. Function pointers pass functions as parameters or store addresses, and are used to implement dynamic calls to functions. Understanding function features and techniques is the key to writing efficient, maintainable, and easy to understand C programs.
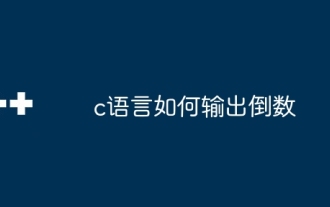
How to output a countdown in C? Answer: Use loop statements. Steps: 1. Define the variable n and store the countdown number to output; 2. Use the while loop to continuously print n until n is less than 1; 3. In the loop body, print out the value of n; 4. At the end of the loop, subtract n by 1 to output the next smaller reciprocal.
