How to Effectively Send and Receive 2D Arrays in MPI?
Sending and Receiving 2D Arrays using MPI
Problem:
You have a large 2D matrix that requires processing across multiple nodes using MPI. The only communication between nodes involves sharing edge values after each time step.
Approach:
Your proposed approach, outlined in the question, is suitable for this problem. The following code segments illustrate the suggested implementation:
// (assume A is a 2D array) if (myrank == 0) { for (i = 0; i < x; i++) { for (j = 0; j < y; j++) { // Perform C++ code implementation MPI_Send(&A[i][0], 3, MPI_INT, 1, 0, MPI_COMM_WORLD); MPI_Recv(&B[0][0], 3, MPI_INT, 1, 0, MPI_COMM_WORLD, &status); MPI_Barrier(MPI_COMM_WORLD); } } } if (myrank == 1) { for (i = x; i < xx; i++) { for (j = 0; j < y; j++) { // Perform C++ code implementation MPI_Send(&B[i][0], 3, MPI_INT, 0, 0, MPI_COMM_WORLD); MPI_Recv(&A[0][0], 3, MPI_INT, 0, 0, MPI_COMM_WORLD, &status); MPI_Barrier(MPI_COMM_WORLD); } } }
Additional MPI Functions:
- MPI_Allgather: Gathers data from all processors into a buffer accessible to all processors. Useful for collecting a distributed array onto a single processor.
- MPI_Alltoall: Provides a complete exchange of data between all processors.
- MPI_Sendrecv: A convenient function that combines a send and receive operation into a single call.
- MPI_Buffer_attach: Attaches a buffer to a process, without needing to explicitly allocate memory for it.
Considerations:
- Contiguous memory allocation is recommended for 2D arrays to simplify MPI communication.
- MPI_Barrier ensures synchronization between processes, but it can be replaced with non-blocking alternatives like MPI_Wait or MPI_Test.
- Blocking sends and receives are used here, but non-blocking options are available for enhanced efficiency.
The above is the detailed content of How to Effectively Send and Receive 2D Arrays in MPI?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
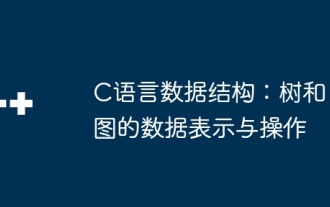
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
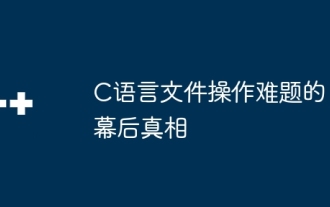
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
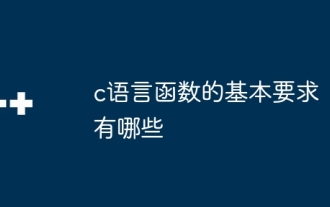
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
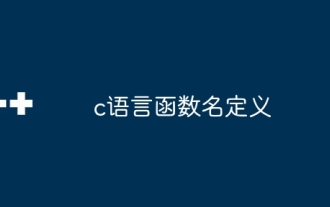
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
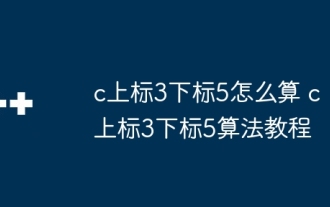
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
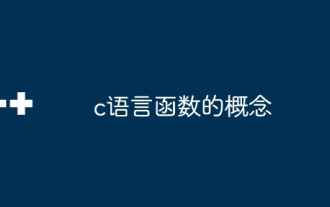
C language functions are reusable code blocks. They receive input, perform operations, and return results, which modularly improves reusability and reduces complexity. The internal mechanism of the function includes parameter passing, function execution, and return values. The entire process involves optimization such as function inline. A good function is written following the principle of single responsibility, small number of parameters, naming specifications, and error handling. Pointers combined with functions can achieve more powerful functions, such as modifying external variable values. Function pointers pass functions as parameters or store addresses, and are used to implement dynamic calls to functions. Understanding function features and techniques is the key to writing efficient, maintainable, and easy to understand C programs.
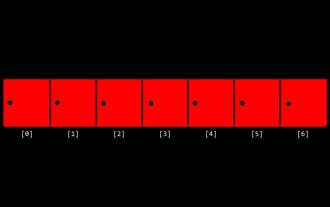
Algorithms are the set of instructions to solve problems, and their execution speed and memory usage vary. In programming, many algorithms are based on data search and sorting. This article will introduce several data retrieval and sorting algorithms. Linear search assumes that there is an array [20,500,10,5,100,1,50] and needs to find the number 50. The linear search algorithm checks each element in the array one by one until the target value is found or the complete array is traversed. The algorithm flowchart is as follows: The pseudo-code for linear search is as follows: Check each element: If the target value is found: Return true Return false C language implementation: #include#includeintmain(void){i
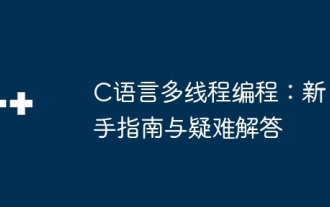
C language multithreading programming guide: Creating threads: Use the pthread_create() function to specify thread ID, properties, and thread functions. Thread synchronization: Prevent data competition through mutexes, semaphores, and conditional variables. Practical case: Use multi-threading to calculate the Fibonacci number, assign tasks to multiple threads and synchronize the results. Troubleshooting: Solve problems such as program crashes, thread stop responses, and performance bottlenecks.
