How Does std::atomic Ensure Data Integrity in Concurrent Programming?
Unveiling the Power of std::atomic
In the realm of concurrent programming, maintaining data integrity across multiple threads is a critical challenge. std::atomic, an integral component of the C Standard Library, offers a solution by providing atomic objects—objects that different threads can simultaneously operate on without inciting undefined behavior.
What does "Atomic Object" Truly Mean?
An atomic object enables simultaneous access from multiple threads, ensuring that each operation (such as read or write) appears to occur instantaneously. This eliminates data races—situations where multiple threads contend to access the same shared data—and ensures the correctness and predictability of concurrent code.
In the example provided, the code snippet:
a = a + 12;
does not constitute a single atomic operation. Instead, it comprises a load of the value of a, the addition of 12 to that value, and a store of the result back to a. Each of these sub-operations is atomic, guaranteeing that the value of a will be modified as intended by each thread.
The = operator, however, provides a genuine atomic operation, equivalent to fetch_add(12, std::memory_order_seq_cst). In this case, the addition is performed atomically, ensuring that the value of a is modified by 12 without the possibility of a data race.
Beyond Atomicity: Memory Ordering and Control
std::atomic empowers programmers with fine-grained control over memory ordering—the sequencing of memory accesses across threads. By specifying memory orders such as std::memory_order_seq_cst or std::memory_order_release, developers can impose explicit synchronization and ordering constraints, ensuring the correct execution of complex concurrent algorithms.
In the code sample below, the "producer" thread generates data and sets the ready_flag to 1 using std::memory_order_release memory order. The "consumer" thread, on the other hand, loads the ready_flag using std::memory_order_acquire memory order. This ensures that the "consumer" thread will only access the data after it has been generated and the ready_flag has been set.
void* sharedData = nullptr; std::atomic<int> ready_flag = 0; // Producer Thread void produce() { sharedData = generateData(); ready_flag.store(1, std::memory_order_release); } // Consumer Thread void consume() { while (ready_flag.load(std::memory_order_acquire) == 0) { std::this_thread::yield(); } assert(sharedData != nullptr); // will never trigger processData(sharedData); }
std::atomic goes beyond mere atomicity, providing comprehensive control over memory access sequencing and synchronization, equipping developers with the tools to create robust and reliable concurrent applications.
The above is the detailed content of How Does std::atomic Ensure Data Integrity in Concurrent Programming?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










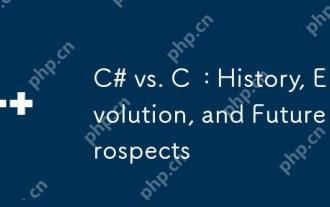
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
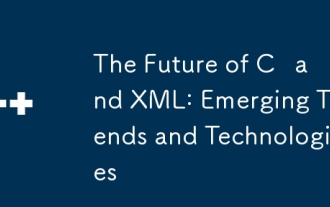
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
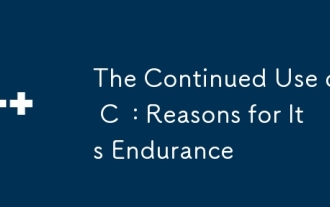
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
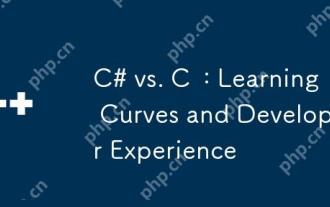
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
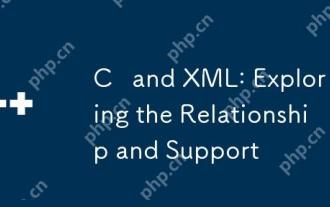
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
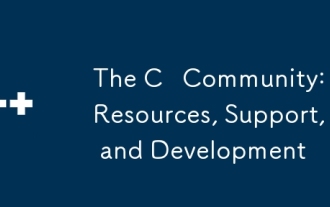
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
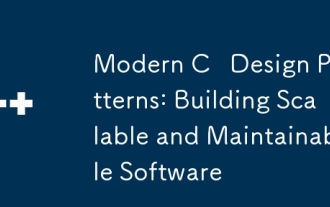
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
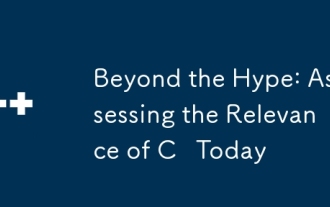
C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
